MQTT-Based LED Control with W55RP20-EVB-Pico: Beebotte IoT Integration Guide
Step-by-step tutorial to connect your W55RP20-EVB-Pico to Beebotte and control LEDs over MQTT.
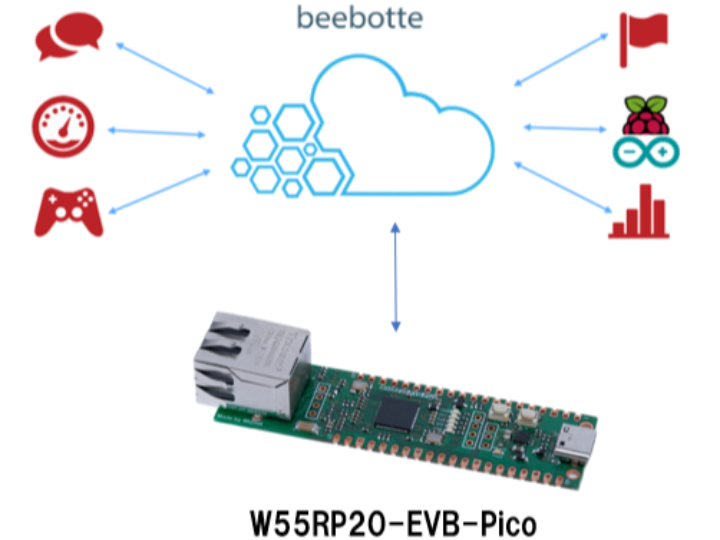
Introduction
In the era of IoT, controlling physical devices through cloud platforms has become a foundational skill. This tutorial demonstrates how to control an onboard LED on the W55RP20-EVB-Pico using Beebotte, a lightweight MQTT-based cloud service designed for real-time IoT applications.
Whether you're an IoT beginner or exploring alternatives to heavyweight cloud platforms like AWS or Azure, this guide walks you through every step — from hardware setup to cloud integration.
Core Hardware & Technology
Component | Description |
---|---|
W55RP20-EVB-Pico | W55RP20-EVB-Pico is an evaluation board for W55RP20, a chip that combines W5500, a wired TCP/IP controller, and RP2040 |
Beebotte | MQTT-based cloud platform for real-time data and device control |
MQTT | Lightweight publish-subscribe messaging protocol ideal for IoT |
LED (Onboard) | Built-in LED on the Pico board for testing control logic |
Implementation
🔌 Hardware Setup
- No additional wiring is required. We use the onboard LED.
- Connect W55RP20-EVB-Pico to your router via Ethernet.
🛠️ Software Prerequisites
- Thonny
- How to build WIZnet-ioNIC-micropython
- WIZnet Ethernet library
- MQTT client library (umqttsimple.py)
☁️ Beebotte Configuration
- Sign up at https://beebotte.com
- Create a channel (e.g.,
W55RP20EVBPico
) - Add a resource (e.g.,
led
) of typeboolean
- Save your Channel Token and Channel Name
- Create a Dashboard
- Add On/Off Widget to Control the LED
🧠 Code Logic
import network
import time
import ujson
import urandom
from machine import Pin, WIZNET_PIO_SPI
from umqtt.simple import MQTTClient
# 핀 설정
LEDPIN = Pin(19, Pin.OUT)
# Beebotte MQTT 설정
BBT = "mqtt.beebotte.com"
TOKEN = "token_"
CHANNEL = "W55RP20EVBPico"
LED_RESOURCE = "led"
# MQTT 클라이언트 생성
client = None
#W5x00 chip init
def w5x00_init():
spi = WIZNET_PIO_SPI(baudrate=31_250_000, mosi=Pin(23),miso=Pin(22),sck=Pin(21)) #W55RP20 PIO_SPI
nic = network.WIZNET5K(spi,Pin(20),Pin(25)) #spi,cs,reset pin
nic.active(True)
#None DHCP
nic.ifconfig(('192.168.11.20','255.255.255.0','192.168.11.1','8.8.8.8'))
#DHCP
#nic.ifconfig('dhcp')
print('IP address :', nic.ifconfig())
while not nic.isconnected():
time.sleep(1)
print(nic.regs())
def generate_id(length=16):
"""랜덤 ID 생성"""
chars = "abcdefghijklmnopqrstuvwxyzABCDEFGHIJKLMNOPQRSTUVWXYZ1234567890"
return ''.join(chars[urandom.getrandbits(6) % len(chars)] for _ in range(length))
def sub_callback(topic, msg):
"""MQTT 메시지 수신 콜백"""
print("Received:", topic, msg)
try:
data = ujson.loads(msg)
led_state = data.get("data", 0)
if led_state:
LEDPIN.on()
else:
LEDPIN.off()
print(f"LED 상태 변경: {'ON' if led_state else 'OFF'}")
except Exception as e:
print("JSON Parsing Error:", e)
def mqtt_connect():
"""MQTT 서버에 연결"""
global client
client = MQTTClient(generate_id(), BBT, 1883, TOKEN, "")
client.set_callback(sub_callback)
try:
client.connect()
topic = f"{CHANNEL}/{LED_RESOURCE}"
client.subscribe(topic)
print(f"Connected to Beebotte MQTT, subscribed to {topic}")
return True
except Exception as e:
print("MQTT 연결 실패:", e)
return False
def main():
"""메인 실행 함수"""
global client
# 이더넷 초기화
w5x00_init()
# MQTT 연결 시도
while not mqtt_connect():
print("MQTT 재연결 중...")
time.sleep(5)
last_reconnect_attempt = time.time()
while True:
try:
client.check_msg() # 새로운 메시지 확인
except Exception as e:
print("MQTT 오류:", e)
# 재연결 로직
if time.time() - last_reconnect_attempt > 5:
print("MQTT 재연결 시도 중...")
last_reconnect_attempt = time.time()
if mqtt_connect():
last_reconnect_attempt = 0
time.sleep(1)
if __name__ == "__main__":
main()