Create an Arduino event driven app on W55RP20-EVB-PICO
Leverage ArduProf library to create a event driven application, running on W55RP20-EVB-PICO.
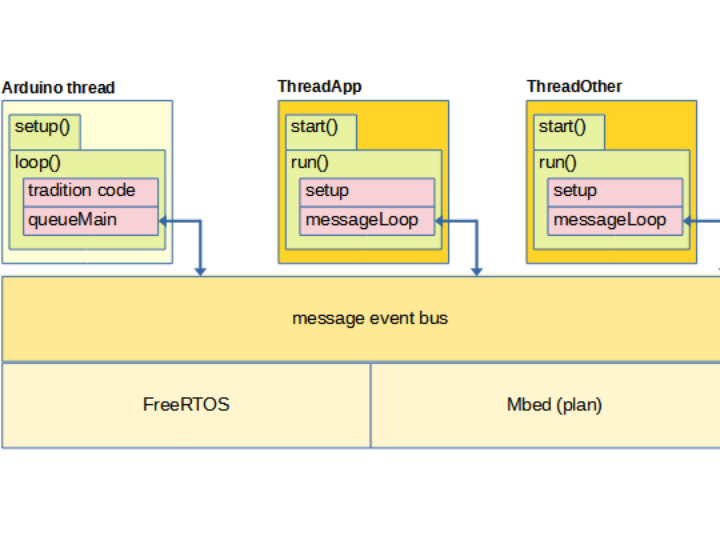
Introduction
Wiznet W55RP20 chip, a System-in-Package (SiP) solution integrates WIZnet's W5500 Ethernet controller with the RP2040 microcontroller from Raspberry Pi, providing networking and processing capabilities for IoT devices and smart applications.
W55RP20-EVB-Pico is an evaluation board for W55RP20, a chip that combines W5500, a wired TCP/IP controller, and RP2040.
This article demonstrates how to create an event driven application for W55RP20, running Arduino Mbed OS.
Step 1: Install Arduino Mbed OS RP2040 Board
Step 2: Install ArduProf library
Step 3: Create an event driven application by clicking "File" -> "Examples" -> "ArduProf" -> "basic"
Step 4: Connect W55RP20-EVB-Pico and PC with a USB cable
Step 5: Select "Raspberry Pi Pico - Arduino Mbed OS RP2040"
Step 6: Run the basic application by clicking "Sketch" -> "Upload"
If everything goes smooth, you should see the followings:
Code explanation
Message format: Format of event message between threads is defined in the file "src/type/Message.h".
It includes fields such as "event" (signed word), "iParam" (signed word), "uParam" (unsigned word) and "lParam" (unsigned double word)
typedef struct _Message
{
int16_t event;
int16_t iParam;
uint16_t uParam;
uint32_t lParam;
} Message;
post event from Arduino loop to ThreadApp
The first step to use ArduProf framework is including the "ArduProf.h" header file. Then defines "queueMain" and "threadApp" and starts them. Posting an event "EventNull" from "queueMain" to "threadApp" is simply as "queueMain.postEvent(context.threadApp, EventNull)"
Sample code is listed in file "basic.ino"
#define ARDUPROF_MBED
#include <ArduProf.h>
// define variable "queueMain" for Arduino thred to interact with ArduProf framework.
static QueueMain queueMain;
// define variable "threadApp" for application thread. (Define other thread as you need)
static ThreadApp threadApp;
// define variable "context" and initialize pointers to "queueMain" and "threadApp"
static AppContext context = {
.queueMain = &queueMain,
.threadApp = &threadApp,
};
void setup()
{
...
// initialize queueMain
queueMain.start(&context);
// start threadApp
threadApp.start(&context);
}
void loop()
{
...
// delay 1s
delay(1000);
// post an event "EventNull" to threadApp
queueMain.postEvent(context.threadApp, EventNull);
}
handling event "EventNull" in ThreadApp
There are 3 steps to handle an event. They are:
1. declare event handler
2. define event handler
3. setup event handler (map event to handler)
Sample code of declare event handler in "ThreadApp.h"
class ThreadApp : public ThreadBase
{
...
///////////////////////////////////////////////////////////////////////
// declare event handler
///////////////////////////////////////////////////////////////////////
__EVENT_FUNC_DECLARATION(EventNull) // void handlerEventNull(const Message &msg);
}
define event handler in "ThreadApp.cpp"
// define EventNull handler
__EVENT_FUNC_DEFINITION(QueueMain, EventNull, msg) // void QueueMain::handlerEventNull(const Message &msg)
{
LOG_TRACE("EventNull(", msg.event, "), iParam=", msg.iParam, ", uParam=", msg.uParam, ", lParam=", msg.lParam);
}
setup event handler (map event to handler) in "ThreadApp.cpp"
ThreadApp::ThreadApp() : ...
{
...
// setup event handlers
handlerMap = {
__EVENT_MAP(ThreadApp, EventNull), // {EventNull, &ThreadApp::handlerEventNull},
};
}
Thanks for your time, hope you enjoy and create a wonderful application on W55RP20.