Real-time GitHub Repository Monitoring with Raspberry Pi Pico and Telegram
Learn how to monitor GitHub repositories using EVB-Pico-W5100. An introductory guide.
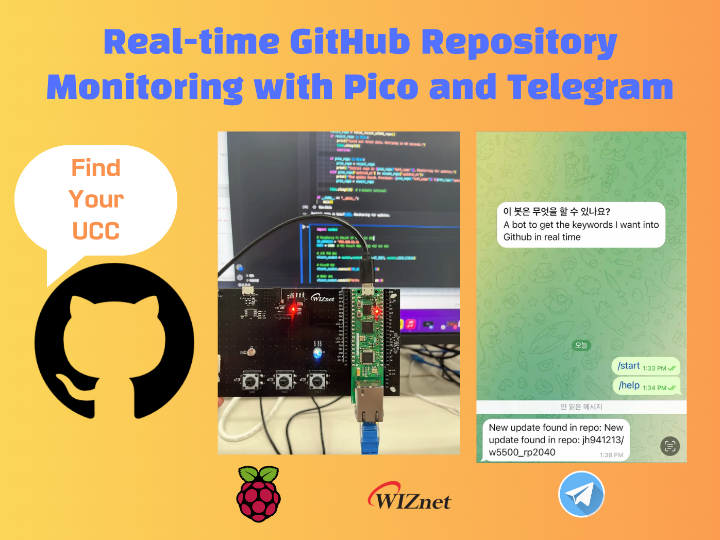
story
In the age of open-source software and collaborative development, keeping track of updates to GitHub repositories has become more crucial than ever. Whether you're a developer, a project manager, or just an enthusiast, you'll find it beneficial to receive real-time updates about your favorite GitHub repositories. That's where the EVB-Pico-W5100 comes in. This project aims to combine the power of Raspberry Pi Pico, a PC server, and Telegram to create a real-time GitHub repository monitoring system.
project purpose
The primary goal of this project is to provide real-time alerts for GitHub repository updates. By leveraging the capabilities of the Raspberry Pi Pico and Telegram's instant messaging service, users can receive immediate notifications whenever a monitored GitHub repository is updated.
Expected Benefits
- Real-time GitHub repository monitoring
- Instant Telegram notifications
- Low-cost solution using Raspberry Pi Pico
- Easy to set up and maintain
- Scalable for monitoring multiple repositories
Requirements
- Raspberry Pi Pico
- Micro USB cable
- Computer with internet access
- Github API Tokens
- Telegram bot
Raspberry Pi Pico Setting
Connect the evb-pico-w5100s to the Ethernet and prepare to communicate with the PC.
- For the firmware and Ethernet communication code related to the RP2040, I referenced the above GitHub to write the code
Basic Examples
#pico test code
from usocket import socket
from machine import Pin,SPI
import network
import time
#W5x00 chip init
def w5x00_init():
spi=SPI(0,2_000_000, mosi=Pin(19),miso=Pin(16),sck=Pin(18))
nic = network.WIZNET5K(spi,Pin(17),Pin(20)) #spi,cs,reset pin
nic.active(True)
#None DHCP
nic.ifconfig(('192.168.1.20','255.255.255.0','192.168.1.1','8.8.8.8'))
#DHCP
#nic.ifconfig('dhcp')
print('IP address :', nic.ifconfig())
while not nic.isconnected():
time.sleep(1)
print(nic.regs())
def server_loop():
s = socket()
s.bind(('192.168.1.20', 5000)) #Source IP Address
s.listen(5)
print("TEST server")
conn, addr = s.accept()
print("Connect to:", conn, "address:", addr)
print("Loopback server Open!")
while True:
data = conn.recv(2048)
print(data.decode('utf-8'))
if data != 'NULL':
conn.send(data)
def main():
w5x00_init()
###TCP SERVER###
#server_loop()
###TCP CLIENT###
client_loop()
if __name__ == "__main__":
main()
Pico Server Code
from usocket import socket
from machine import Pin, SPI
import network
import time
import urequests # MicroPython requests library
# Telegram Bot Token
TELEGRAM_TOKEN = "6552216859:AAGqDyzbGkxP6S7mEAbMdYsp-6BJX02DWtc"
CHAT_ID = "5795970252"
# Send message to Telegram
def send_telegram_message(message):
url = f"https://api.telegram.org/bot{TELEGRAM_TOKEN}/sendMessage"
data = {
"chat_id": CHAT_ID,
"text": message
}
urequests.post(url, json=data)
#W5x00 chip init
def w5x00_init():
spi=SPI(0,2_000_000, mosi=Pin(19),miso=Pin(16),sck=Pin(18))
nic = network.WIZNET5K(spi,Pin(17),Pin(20)) #spi,cs,reset pin
nic.active(True)
#None DHCP
nic.ifconfig(('Your IP','255.255.255.0','Your IP gateway','8.8.8.8'))
#DHCP
#nic.ifconfig('dhcp')
print('IP address :', nic.ifconfig())
while not nic.isconnected():
time.sleep(1)
print(nic.regs())
def server_loop():
s = socket()
s.bind(('Your IP', 5000))
s.listen(5)
print("TEST server")
conn, addr = s.accept()
print("Connect to:", conn, "address:", addr)
print("Loopback server Open!")
while True:
data = conn.recv(2048)
repo_info = data.decode('utf-8')
print(repo_info)
if repo_info != 'NULL':
conn.send(data)
send_telegram_message(f"New update found in repo: {repo_info}")
def main():
w5x00_init()
server_loop()
if __name__ == "__main__":
main()
PC Server
GitHub API
1. Log in to Github and tap Settings.
2.On the Your personal account screen, tap Devpoer settings
3.Tap Generate classic token.
4. Select the Scopes you want to call with the API and create a token.
Basic Examples
#Code to send from PC server to Pico
import socket
IP_ADDRESS = 'Your Server IP'
PORT = 5000
client_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
client_socket.connect((IP_ADDRESS, PORT))
client_socket.sendall("Hello Pico".encode())
data = client_socket.recv(1024)
print('Received:', data.decode())
PC GitHub Monitoring and Sending Code
import requests
import time
# GitHub Personal Access Token
TOKEN = "Your GitHub Token"
# GitHub API URL for searching repositories
SEARCH_API_URL = "https://api.github.com/search/repositories"
def fetch_recent_w5500_repo():
headers = {
'Authorization': f'token {TOKEN}',
'Accept': 'application/vnd.github.v3+json',
'User-Agent': 'My-App'
}
params = {
'q': 'W5500', #your keyword
'sort': 'updated',
'order': 'desc'
}
response = requests.get(SEARCH_API_URL, headers=headers, params=params)
if response.status_code == 200:
data = response.json()
if data.get('items'):
return data['items'][0]
else:
print(f"Error: {response.content}")
return None
def main():
prev_repo = None
while True:
recent_repo = fetch_recent_w5500_repo()
if recent_repo is None:
print("Could not fetch data. Retrying in 60 seconds.")
time.sleep(60)
continue
if prev_repo is None:
prev_repo = recent_repo
print(f"Initial repo is {prev_repo['full_name']}. Monitoring for updates.")
elif prev_repo['updated_at'] != recent_repo['updated_at']:
print(f"New update found. Previous: {prev_repo['full_name']} ({prev_repo['updated_at']}), Current: {recent_repo['full_name']} ({recent_repo['updated_at']})")
prev_repo = recent_repo
time.sleep(10) # 10seconds interval
if __name__ == "__main__":
main()
steps
- Install Dependencies: Install the required Python packages using pip.
- Run Server Code: Execute the provided Python script to start the server.
- Test Connection: Make sure the server can communicate with the Raspberry Pi Pico and fetch data from GitHub.
Telegram
Telegram Bot make
# Telegram Bot Token
TELEGRAM_TOKEN = "Your Token"
CHAT_ID = "Your ID"
# Send message to Telegram
def send_telegram_message(message):
url = f"https://api.telegram.org/bot{TELEGRAM_TOKEN}/sendMessage"
data = {
"chat_id": CHAT_ID,
"text": message
}
urequests.post(url, json=data)
steps
- Create a Bot: Use the Telegram BotFather to create a new bot.
- Get Token: Obtain the API token for your bot.
- Configure Code: Update the Raspberry Pi Pico code with your Telegram bot's API token.
- Test Notifications: Ensure that you can receive Telegram notifications from the Raspberry Pi Pico.
Communication
The Raspberry Pi Pico and the PC server communicate over a local network. The server fetches the latest updates from GitHub and sends this information to the Raspberry Pi Pico. The Pico then forwards these updates to Telegram.
Result
Upon successful setup, you'll start receiving real-time Telegram notifications whenever there's an update to the monitored GitHub repository. This project offers a cost-effective and straightforward way to stay updated on your favorite GitHub projects.
Feel free to expand upon this project by adding more features, such as monitoring multiple repositories or integrating with other messaging platforms.
Discover open source repository treasures together with Pico
For more code
https://github.com/wiznetmaker maker site github
https://github.com/jh941213 simon github