Modbus_MQTT_module
This project is a FreeRTOS-based system that utilizes MQTT and ModbusTCP protocols for network-based device control and data communication.
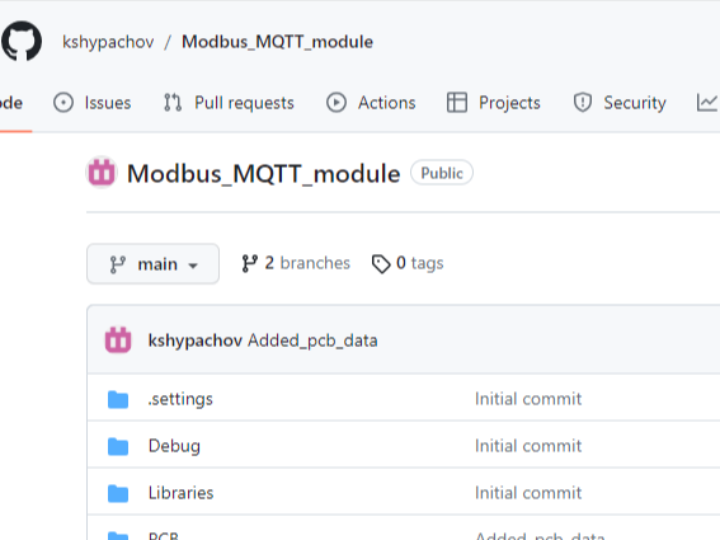
Overview
This README provides documentation for the code focused on MQTT and Modbus functionality. It is designed to be a part of a larger system that utilizes both MQTT for messaging and Modbus over TCP for industrial automation.
MQTT Functionality
Description
MQTT (Message Queuing Telemetry Transport) is a lightweight messaging protocol for small sensors and mobile devices. It is optimized for high-latency or unreliable networks.
Example Usage
Initialization and Configuration:
- Initialize the MQTT credentials.
- Set default parameters if MQTT credentials are not found.
- Open and read the MQTT credentials file using lfs_file_opencfg, lfs_file_read, and lfs_file_close.
MQTT Communication:
- Check and update MQTT credentials periodically.
- Write the updated credentials back to the file system.
- Use MQTT for various tasks such as sending sensor data or receiving commands.
Key Functions
- vRead_and_write_settings: Initializes and mounts the file system, reads and writes MQTT and Modbus settings.
- vInternet_MQTT: Handles the MQTT protocol including connection, subscription, and data exchange.
Modbus Functionality
Description
Modbus is a communication protocol for industrial devices. Modbus TCP is a variant of the Modbus protocol used over TCP/IP networks.
Example Usage
Initialization and Configuration:
- Initialize Modbus TCP settings.
- Set default parameters if Modbus TCP settings are not found.
- Open and read the Modbus TCP settings file using lfs_file_opencfg, lfs_file_read, and lfs_file_close.
Modbus TCP Operations:
- Handle Modbus TCP requests and responses.
- Update Modbus TCP settings and write back to the file system when needed.
Key Functions
- vRead_and_write_settings: Reads and writes Modbus TCP settings from/to the file system.
void vRead_and_write_settings(void *pvParameters) {
// Initialize MQTT credentials structure
MQTT_cred_struct MQTT_credentials;
// Initialize and mount the file system
io_fs_init(lfs_read_buf, lfs_write_buf, lfs_lookahead_buff, LFS_BUF_SIZE);
lfs_mount(&lfs, &cfg);
// Check and read MQTT credentials file
if ((lfs_stat(&lfs, "MQTT_credentials", &file_info) >= 0) && (file_info.size != 0)) {
lfs_file_opencfg(&lfs, &file, "MQTT_credentials", LFS_O_RDONLY, &fileConf);
lfs_file_read(&lfs, &file, &MQTT_credentials, sizeof(MQTT_credentials));
lfs_file_close(&lfs, &file);
}
// Other initialization and setup code...
}
- vInternetModbusTCP: Manages Modbus TCP connections and handles requests.
void vInternetModbusTCP(void *pvParameters) {
// Initialize Modbus TCP structure
ModBusTCP_struct ModBusTCP_parameters;
// Initialize Ethernet info structure
Ethernet_info_struct EthernetInfo;
while (1) {
xQueuePeek(EthernetInfoQ, &EthernetInfo, 0);
// Check for Ethernet connection
if (EthernetInfo.link == ETH_LINK_UP && assigned_ip()) {
xSemaphoreTake(SocketMutex, portMAX_DELAY);
if (eMBTCPInit(M
General Workflow
- Initialization: The main function initializes system configurations and creates various tasks including MQTT and Modbus.
- Task Execution: The MQTT and Modbus tasks (vInternet_MQTT and vInternetModbusTCP) are executed in parallel with other tasks, handling their respective protocols.
- Data Handling: Both protocols involve reading and writing configuration data to a LittleFS file system and communicating over network interfaces.
MQTT and Network-Related Features and Functions
MQTT and Network-Related Features
- void vApplicationTickHook(void): This function is called with every system timer tick and executes mqtt_1ms_tik() for managing MQTT communication timers.
- vRead_and_write_settings(void *pvParameters): This function is responsible for reading and writing system settings. It handles reading and writing MQTT settings (MQTT_cred_struct) and ModbusTCP settings (ModBusTCP_struct) from the file system.
- vInternetMainTask(void *pvParameters): This function performs tasks required for internet connectivity, including DHCP and network status management.
- vInternetModbusTCP(void *pvParameters): This function manages data communication using the ModbusTCP protocol.
- vInternetHTTPd(void *pvParameters): This function operates and manages an HTTP server.
- vInternet_MQTT(void *pvParameters): This function involves sending and receiving data using the MQTT protocol.
MQTT and Network-Related Data Structures
- MQTT_cred_struct: A structure that stores configuration information needed for MQTT connections, such as IP, port, login details.
- ModBusTCP_struct: A structure for ModbusTCP protocol settings.
- Ethernet_info_struct: A structure that stores network state information (IP, MAC address, etc.).
Key FreeRTOS Components
- Creation of Queues (xQueueCreate), Semaphores (xSemaphoreCreateMutex), and Tasks (xTaskCreate): These elements are used for resource management, task synchronization, and communication in a multitasking environment.
Main FreeRTOS Functions
- vTaskDelay: Delays the execution of a task for a specified time.
- xQueueOverwrite, xQueuePeek: Writes to or reads from a queue.
- xSemaphoreTake, xSemaphoreGive: Controls access to resources using semaphores.
Network Communication Related Functions
- w5500_lib_init, reg_wizchip_spi_cbfunc, DHCP_init: Functions for initializing and configuring the W5500 network chip.
This code performs various network-based functionalities including network settings, MQTT, ModbusTCP protocol for data exchange, and web server operations. It utilizes FreeRTOS queues, semaphores, and tasks for resource management and synchronization in a multitasking environment.
Modbus Protocol for Device Control
The Modbus protocol is primarily used in industrial automation and control systems. It employs a simple yet powerful message structure to facilitate communication between controllers and devices. Modbus supports two main modes, Modbus ASCII and Modbus RTU, and can be extended over networks via TCP/IP.
Key Features of Modbus-Controlled Operations:
Registers and Coils:
- Modbus protocol uses two fundamental data types, "Registers" and "Coils", for transmitting various kinds of data.
- Coils: Represent digital values (ON/OFF) and are used to control digital outputs of a device.
- Registers: Represent analog values or larger data blocks and are used for transmitting information like sensor readings or settings.
Communication Method:
- Modbus operates on a master/slave communication model. The master (usually a controller) sends requests to slaves (sensors, actuators, etc.), and slaves respond to these requests.
- Each slave device has a unique address.
Key Commands:
- Read Commands: For reading the status of coils or registers, such as 'Read Coils' or 'Read Holding Registers'.
- Write Commands: For changing the status of coils or registers, for example, 'Write Single Coil' or 'Write Multiple Registers'.
Application Cases:
- Modbus protocol is used to control various devices in industrial settings, such as PLCs, sensors, valves, and motors.
- Tasks such as reading values from specific sensors, starting or stopping motors, or changing settings of temperature control devices can be performed.
Error Handling:
- The Modbus protocol includes the capability to detect communication errors and return error codes.
Example Scenario:
For instance, in Modbus device control, the following scenarios can occur:
- The master (controller) uses the 'Read Holding Registers' command to read temperature values from a specific sensor.
- The master uses the 'Write Single Coil' command to turn a pump on or off.
- The master changes several settings of a device simultaneously using the 'Write Multiple Registers' command.