8. MicroPython development for W5100S/W5500+RP2040<HTTP Server example>
8. MicroPython development for W5100S/W5500+RP2040<HTTP Server example>
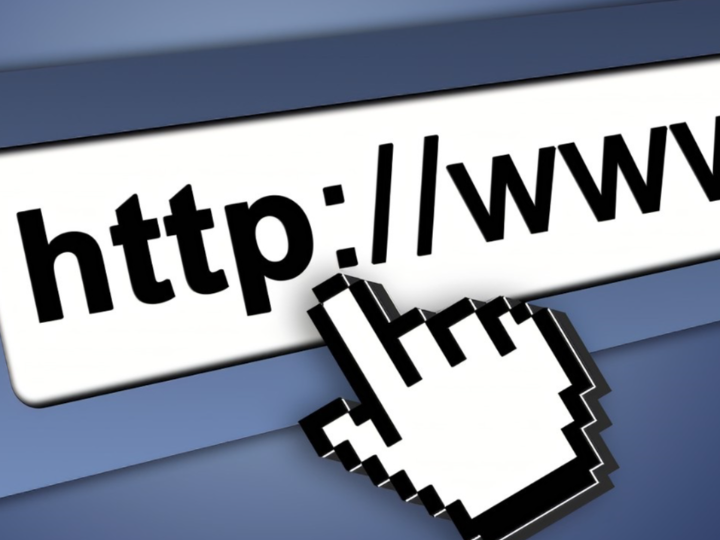
1 Introduction
With the promotion and popularity of cloud computing, more and more network devices and services need to be connected to the network, which means that more IP addresses and other network configuration information are required. The DHCP server can dynamically allocate IP addresses and other configuration information, simplifying network configuration management and improving the availability and efficiency of network equipment.
W5100S/W5500 is an embedded Ethernet controller integrating a full hardware TCP/IP protocol stack. It is also an industrial-grade Ethernet control chip. This tutorial will introduce the basic principles, usage steps, application examples and precautions of W5100S/W5500 Ethernet HTTP Server application to help readers better master this technology.
2. Related network information
2.1 Introduction
HTTP, the full name of Hypertext Transfer Protocol, is the most widely used network transmission protocol. It is a transmission protocol used to transmit hypertext from the World Wide Web server to the local browser. All WWW files must comply with this standard. It is a communication protocol based on TCP/IP to transfer data (HTML files, image files, query results, etc.).
2.2 HTTP Server working steps
The principle steps of HTTP Server can be summarized as the following points:
Establish a connection: The web browser establishes a connection with the web server and opens a virtual file called socket. The establishment of this file indicates that the connection is successfully established.
Request: The web browser submits a request to the web server through the socket. HTTP requests are generally GET or POST commands (POST is used to pass FORM parameters).
The server processes the request: Once the request is received, the server finds the corresponding resource based on the request type and URL and reads the file content. If it is a POST request, the server will also parse the form data.
Response: The server returns the processed data to the client through the HTTP protocol, including HTTP header information and HTML file content.
Close the connection: The connection between the client and the server is closed after the data exchange is completed.
2.3 Advantages of HTTP Server
The advantages of HTTP Server include:
Simple and Flexible: The HTTP protocol is simple, easy to understand and implement, so developers can build and deploy applications faster.
Easy to extend: The HTTP protocol supports a variety of extensions, making it easy to add new functions and features to meet changing needs.
Stateless: The HTTP protocol is stateless, which means the server does not maintain state for every request. While this may cause some problems, statelessness also makes the HTTP protocol more flexible and scalable.
Cross-platform support: The HTTP protocol is a universal network protocol that can be implemented and used on a variety of operating systems and devices.
Supports multiple programming languages: The HTTP protocol does not limit the programming language used, so applications can be developed and deployed using various programming languages.
Good maintainability: The HTTP protocol specification is clear, easy to understand and maintain, and there are many mature tools and libraries that allow developers to build and deploy applications faster.
Support multimedia content: The HTTP protocol can transmit many types of data, including multimedia content such as text, pictures, audio, and video, allowing developers to easily build multimedia applications.
Support caching and compression: The HTTP protocol supports caching and compression technology, which can reduce the amount of data transmitted over the network and improve application performance and response speed.
2.4 HTTP Server application scenarios
The application scenarios of HTTP Server are very wide. The following are some main scenarios:
File Sharing: The most widespread use of HTTP Server is to allow file sharing on the Internet. By using HTTP Server, users can download or upload files from the server.
Web applications: Most websites and applications are inseparable from HTTP Server. HTTP Server can process the client's request and return the processing result to the client.
Cloud services: Many cloud services rely on HTTP Server, such as Amazon's S3, Google Cloud Storage, etc. These services provide a RESTful API that uses HTTP for interaction.
Big data: Big data tools such as Hadoop and Spark all support access and processing of data through HTTP Server.
Internet of Things: In the field of Internet of Things, HTTP Server also plays a big role. For example, many smart home devices use HTTP Server to interact with mobile applications or web pages.
Streaming media: HTTP Server is also widely used for streaming media services, such as some online video websites and music websites.
Game development: Some web-based games also use HTTP Server for communication between client and server.
Remote management: The remote management interface of many devices is a simple Web page that accepts user input and returns results through HTTP Server.
3 WIZnet Ethernet chip
WIZnet mainstream hardware protocol stack Ethernet chip parameter comparison
Model | Embedded Core | Host I/F | TX/RX Buffer | HW Socket | Network Performance |
---|---|---|---|---|---|
W5100S | TCP/IPv4, MAC & PHY | 8bit BUS, SPI | 16KB | 4 | Max.25Mbps |
W6100 | TCP/IPv4/IPv6, MAC & PHY | 8bit BUS, Fast SPI | 32KB | 8 | Max.25Mbps |
W5500 | TCP/IPv4, MAC & PHY | Fast SPI | 32KB | 8 | Max.15Mbps |
W5100S/W6100 supports 8-bit data bus interface, and the network transmission speed will be better than W5500.
W6100 supports IPv6 and is compatible with W5100S hardware. If users who already use W5100S need to support IPv6, they can be Pin to Pin compatible.
W5500 has more Sockets and send and receive buffers than W5100S.
4 HTTP Network Settings Example Overview and Usage
4.1 Flowchart
The running block diagram of the program is as follows:
4.2 Core preparation work
Software
Thonny
Hardware
W5100SIO module + RP2040 Raspberry Pi Pico development board or Wiznet W5100S-EVB-Pico development board
Micro USB interface data cable
cable
4.3 Connection method
Connect the USB port of the PC through the data cable (mainly used for burning programs, but can also be used as a virtual serial port)
When wiring using module connection RP2040
RP2040 GPIO16 <----> W5100S MISO
RP2040 GPIO17 <----> W5100S CS
RP2040 GPIO18 <----> W5100S SCK
RP2040 GPIO19 <----> W5100S MOSI
RP2040 GPIO20 <----> W5100S RST
Connect the PC and device to the router LAN port through network cables
4.4 Main code overview
We open the http_server.py file directly. Step one: You can see that SPI is initialized in the w5x00_init() function. And register the SPI related pins and reset pins into the library. The subsequent step is to activate the network and use DHCP to configure the network address information. When DHCP fails, configure the static network address information. When the configuration is not successful, the information about the network address-related registers will be printed out, which can help us better troubleshoot the problem.
Step 2: Set the socket number and bind the server IP and port number.
Step 3: Listen and wait for client connection.
Step 4: Set up the web interface to parse the client's request to control the light switch. At the same time, the window prints out the status of the light and the client’s IP information.
''' HTTP Server example.
date: 2023-11-27
'''
from usocket import socket
from machine import Pin,SPI
import time, network
''' LED init
'''
led = Pin(25, Pin.OUT)
''' static netinfo
'''
ip = '192.168.1.11'
sn = '255.255.255.0'
gw = '192.168.1.1'
dns= '8.8.8.8'
netinfo=(ip, sn, gw, dns)
localip = ''
localport = 8000
listen_info = (localip, localport)
def w5x00_init():
global localip
''' spi0 init
baudrate: 2000000
mosi pin: gpio19
miso pin: gpio16
sck pin: gpio18
cs pin: gpio17
rst pin: gpio20
'''
spi=SPI(0,2_000_000, mosi=Pin(19),miso=Pin(16),sck=Pin(18))
nic = network.WIZNET5K(spi,Pin(17),Pin(20))
nic.active(True)
# use dhcp, if fail use static netinfo
try:
nic.ifconfig('dhcp')
except:
nic.ifconfig(netinfo)
localip = nic.ifconfig()[0]
print('ip :', nic.ifconfig()[0])
print('sn :', nic.ifconfig()[1])
print('gw :', nic.ifconfig()[2])
print('dns:', nic.ifconfig()[3])
while not nic.isconnected():
time.sleep(1)
# print(nic.regs())
print('no link')
uart.write('no link\r\n')
''' web page info
'''
def web_page():
if led.value()==1:
led_state="ON"
else:
led_state="OFF"
html = """
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta http-equiv="X-UA-Compatible" content="IE=edge">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Raspberry Pi Pico Web server - WIZnet W5100S</title>
</head>
<body>
<div align="center">
<H1>Raspberry Pi Pico Web server & WIZnet Ethernet HAT</H1>
<h2>Control LED</h2>
PICO LED state: <strong>""" + led_state + """</strong>
<p><a href="/?led=on"><button class="button">ON</button></a><br>
</p>
<p><a href="/?led=off"><button class="button button2">OFF</button></a><br>
</p>
</div>
</body>
</html>
"""
return html
def main():
global localip
w5x00_init()
s = socket()
s.bind((localip, 80))
s.listen(5)
while True:
conn, addr = s.accept()
print('Connect from %s' % str(addr))
request = conn.recv(1024)
request = str(request)
#print('Content = %s' % request)
led_on = request.find('/?led=on')
led_off = request.find('/?led=off')
if led_on == 6:
print("LED ON")
led.value(1)
if led_off == 6:
print("LED OFF")
led.value(0)
response = web_page()
conn.send('HTTP/1.1 200 OK\n')
conn.send('Connection: close\n')
conn.send('Content-Type: text/html\n')
conn.send('Content-Length: %s\n\n' % len(response))
conn.send(response)
conn.close()
if __name__ == "__main__":
main()
4.5 Results demonstration
Step 1: Open thonny, select the environment as Raspberry Pi Pico, copy the program to Thonny, and then click Run.
Step 2: Open the browser and enter the server IP to enter the web page.
Step 3: Select the status of the light to control. After clicking on the browser interface to control the status of the light, the window on Thonny will print the status of the light and the client IP.
Note: Because MicroPython's print function enables stdout buffering, sometimes the content will not be printed out immediately.
5 Precautions
If you use Wiznet's W5500 to implement the examples in this chapter, you only need to burn the firmware of the W5500 and run the example program.