7. MicroPython development for W5100S/W5500+RP2040<HTTP Client example>
7. MicroPython development for W5100S/W5500+RP2040<HTTP Client example>
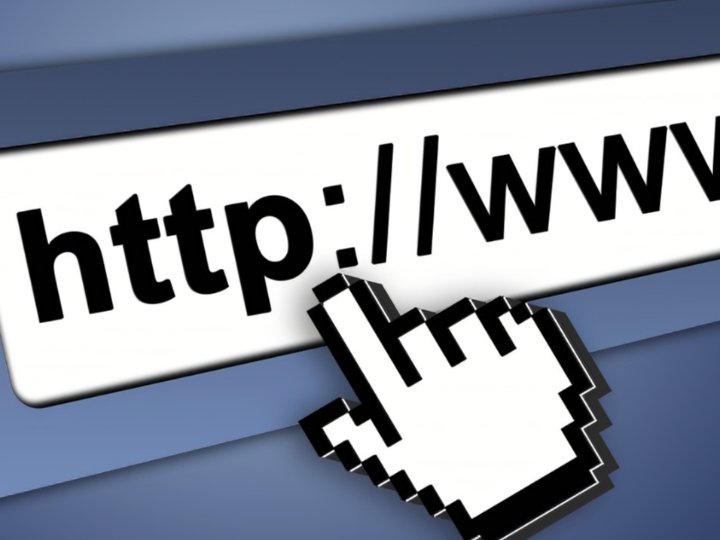
In this era of smart hardware and the Internet of Things, MicroPython and Raspberry Pi PICO are leading the new trend of embedded development with their unique advantages. MicroPython, as a streamlined and optimized Python 3 language, provides efficient development and easy debugging for microcontrollers and embedded devices.
When we combine it with the WIZnet W5100S/W5500 network module, the development potential of MicroPython and Raspberry Pi PICO is further amplified. Both modules have built-in TCP/IP protocol stacks, making it easier to implement network connections on embedded devices. Whether it is data transmission, remote control, or building IoT applications, they provide powerful support.
In this chapter, we will take WIZnet W5100S as an example to use MicroPython development method to perform GET and POST request examples in HTTP Client mode.
2. Related network information
2.1 Introduction
The HTTP protocol is a hypertext transfer protocol that specifies the communication rules between the client and the server and is used to transmit data on the network. The HTTP protocol communicates based on the TCP/IP protocol suite and is an application layer protocol.
HTTP Client is the client mode of HTTP protocol.
2.2 Principle
In HTTP client mode, two commonly used request methods are GET and POST. Their differences are as follows:
Data transmission method: The GET method includes parameters in the URL, while the POST method passes parameters through the request body.
Data Visibility: The parameters of the GET method are visible to everyone in the URL, while the data of the POST method will not be displayed in the URL.
Data length: The parameter length of the GET method is limited, while the POST method has no limit on the data length.
Data type: The GET method only accepts ASCII characters, while the POST method has no restrictions and also allows binary data.
Security: The POST method is more secure than the GET method because the parameters are not saved in the browser history or web server logs.
Caching: GET requests will be actively cached by the browser, while POST requests will not unless set manually.
History: GET request parameters will be completely retained in the browser history, while parameters in POST will not be retained.
Bookmarks: The URL address generated by GET can be bookmarked, but POST cannot.
Here's how they request data:
2.3 Request method
In addition, there are the following request methods
HEAD: Similar to a GET request, except that there is no specific content in the returned response, used to obtain headers.
PUT: Data transferred from the client to the server replaces the contents of the specified document.
DELETE: Request the server to delete the specified page.
CONNECT: The HTTP/1.1 protocol is reserved for proxy servers that can change the connection to a pipeline.
OPTIONS: Allows clients to view server performance.
TRACE: Echo the request received by the server, mainly used for testing or diagnosis.
PATCH: It is a supplement to the PUT method and is used to locally update known resources.
2.4 Application
In the field of Internet of Things (IoT), the client mode of the HTTP protocol has many applications.
Device Control: IoT devices, such as smart home devices (light bulbs, thermostats, etc.), can receive commands from the server through HTTP client mode to change the device state.
Data upload: IoT devices, such as sensors, can send collected data to a server using the HTTP POST method. This data can include temperature readings, humidity readings, motion detection data, and more.
Device updates: IoT devices can download updates from the server using the HTTP GET method. This can include firmware updates, configuration updates, etc.
Status synchronization: IoT devices can periodically send their status to the server using HTTP GET or POST methods for remote monitoring or troubleshooting.
Service Integration: IoT devices can be integrated with various online services using HTTP client mode. For example, a smart thermostat can use the HTTP GET method to obtain weather forecast data to adjust the indoor temperature more effectively.
3. WIZnet Ethernet chip
WIZnet mainstream hardware protocol stack Ethernet chip parameter comparison
Model | Embedded Core | Host I/F | TX/RX Buffer | HW Socket | Network Performance |
---|---|---|---|---|---|
W5100S | TCP/IPv4, MAC & PHY | 8bit BUS, SPI | 16KB | 4 | Max 25Mbps |
W6100 | TCP/IPv4/IPv6, MAC & PHY | 8bit BUS, Fast SPI | 32KB | 8 | Max 25Mbps |
W5500 | TCP/IPv4, MAC & PHY | Fast SPI | 32KB | 8 | Max 15Mbps |
W5100S/W6100 supports 8-bit data bus interface, and the network transmission speed will be better than W5500.
W6100 supports IPv6 and is compatible with W5100S hardware. If users who already use W5100S need to support IPv6, they can be Pin to Pin compatible.
W5500 has more Sockets and send and receive buffers than W5100S
Compared with the software protocol stack, WIZnet's hardware protocol stack Ethernet chip has the following advantages:
Hardware TCP/IP protocol stack: WIZnet's hardware protocol stack chip provides a hardware-implemented TCP/IP protocol stack. This hardware-implemented protocol stack has better performance and stability than software-implemented protocol stacks.
No additional embedded system software stack and memory resources required: Since all Ethernet transmit and receive operations are handled by the independent Ethernet controller, no additional embedded system software stack and memory resources are required.
Resistant to network environment changes and DDoS attacks: Compared with software TCP/IP protocol stacks that are susceptible to network environment changes and DDoS attacks, hardware protocol stack chips can provide more stable Ethernet performance.
Suitable for low-specification embedded systems: Even in low-specification embedded systems, hardware protocol stack chips using WIZnet can show more efficient Internet application operating performance than high-specification systems using software TCP/IP protocol stacks.
4. HTTP Client communication example explanation and use
4.1 Program flow chart
4.2 Test preparation
Software:
Thonny
Hardware:
W5100S IO module + RP2040 Raspberry Pi Pico development board or WIZnet W5100S-EVB-Pico development board
Micro USB interface data cable
cable
4.3 Connection method
Connect to PC USB port via data cable
When using W5100S/W5500 IO module to connect to RP2040
RP2040 GPIO 16 <----> W5100S/W5500 MISO
RP2040 GPIO 17 <----> W5100S/W5500 CS
RP2040 GPIO 18 <----> W5100S/W5500 SCK
RP2040 GPIO 19 <----> W5100S/W5500 MOSI
RP2040 GPIO 20 <----> W5100S/W5500 RST
Directly connect to the PC network port through a network cable (or: both the PC and the device are connected to the switch or router LAN port through a network cable)
4.4 Related code
We open the http_client.py file directly.
Step one: You can see that SPI is initialized in the w5x00_init() function. And register the spi-related pins and reset pins into the library. The subsequent step is to activate the network and use DHCP to configure the network address information. When DHCP fails, configure the static network address information. When the configuration is not successful, the information about the network address-related registers will be printed out, which can help us better troubleshoot the problem.
Step 2: In the request() function, perform GET and POST request tests.
''' HTTP Client example.
date: 2023-11-27
'''
from usocket import socket
from machine import Pin,SPI,UART
import time, network
import urequests
''' static netinfo
'''
ip = '192.168.1.11'
sn = '255.255.255.0'
gw = '192.168.1.1'
dns= '8.8.8.8'
netinfo=(ip, sn, gw, dns)
geturl = "http://httpbin.org/get"
posturl = "http://httpbin.org/post"
def w5x00_init():
global localip
spi=SPI(0,2_000_000, mosi=Pin(19),miso=Pin(16),sck=Pin(18))
nic = network.WIZNET5K(spi,Pin(17),Pin(20))
nic.active(True)
try:
#DHCP
print("\r\nConfiguring DHCP")
nic.ifconfig('dhcp')
except:
#None DHCP
print("\r\nDHCP fails, use static configuration")
nic.ifconfig(('192.168.1.20','255.255.255.0','192.168.1.1','8.8.8.8'))#Set static network address information
#Print network address information
print("IP :",nic.ifconfig()[0])
print("Subnet Mask:",nic.ifconfig()[1])
print("Gateway :",nic.ifconfig()[2])
print("DNS :",nic.ifconfig()[3],"\r\n")
#If there is no network connection, the register address information is printed
while not nic.isconnected():
time.sleep(1)
print(nic.regs())
def request():
print("GET Request test")
r = urequests.get(geturl+"?WIZnet=W5100S_W5500")
print("Request response code:",r.status_code)
print("Request response args:",r.json()["args"])
r= urequests.post(posturl ,json={"WIZnet":"W5100S_W5500"})
if not r:
print('spreadsheet: no response received')
print("\r\nPOST Request test")
print("Request response code:",r.status_code)
print("Request response data:",r.json()["data"])
def main():
w5x00_init()
request()
if __name__ == "__main__":
main()
4.5 Verification
To test the Ethernet examples, the development environment must be configured to use a Raspberry Pi Pico.
Required development environment
If you must compile MicroPython, you must use a Linux or Unix environment.
Step 1: Select the environment as Raspberry Pi Pico and save the urequests.py library file to the Raspberry Pi.
Step 2: Copy the program to Thonny, and then click Run. You can see that the response codes and submitted data contents of the GET request and POST request are printed out respectively.
Note: Because MicroPython's print function enables stdout buffering, sometimes the content will not be printed out immediately.
5. Precautions
If you use WIZnet's W5500 to implement the examples in this chapter, you only need to burn the firmware of the W5500 and run the example program.