31. W5100S/W5500+RP2040 Raspberry Pi Pico<TCP_Server multi-socket>
31. W5100S/W5500+RP2040 Raspberry Pi Pico<TCP_Server multi-socket>
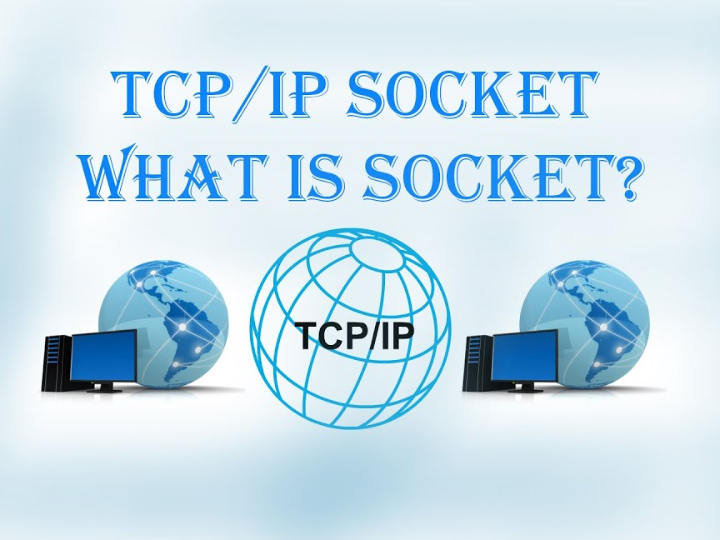
1 Introduction
W5100S/W5500 is an embedded Ethernet controller that integrates a full hardware TCP/IP protocol stack. It is also an industrial-grade Ethernet control chip. Supports independent communication through multiple sockets, and communication efficiency does not affect each other. This tutorial will introduce the basic principles, usage steps, application examples and precautions of W5100S/W5500 Ethernet multi-socket application to help readers better master this technology.
2. 1 Advantages of using multiple sockets
The advantages of multi-channel sockets are mainly reflected in the following aspects:
Improve concurrent processing capabilities: Multiple sockets can handle multiple network connection requests at the same time, significantly improving the server's performance and concurrent processing capabilities. This means that the server can handle requests from multiple clients at the same time without creating a thread for each client connection.
Enhanced reliability: Multi-channel sockets can provide connections between the main server and the backup server for scenarios with high reliability requirements. In actual use, when an exception occurs on the primary server, services can be provided through the backup server.
Optimized data processing: Multiple sockets can be optimized according to different network connection requests. For example, for connections with long data transmission intervals, network heartbeat packets are used to regularly upload a set of fixed data to let the server know that the device is in normal working condition; for triggering In the scenario of uploading data in a formal way, unnecessary processing can be reduced.
Convenient device identification: When the device starts, multiple sockets can upload a set of information with a unique number to the server, which is used by the server to identify which device it is and facilitate subsequent data interaction.
2.2 Principle of multi-channel socket data interaction
The process for the server to establish multiple sockets can be carried out according to the following steps:
The server creates a socket and binds it to an IP address and a port, then starts listening and waits for the client's connection request.
When the server receives the client's connection request, it will create a new socket for the connection and establish a connection with the client.
The server and client can exchange data through their respective sockets.
When the data interaction is completed, the connection can be closed (maybe actively or passively).
The server continues to listen, waiting for the next client connection request.
2.3 Multi-channel socket application scenarios
Multi-socket applications refer to applications that use socket interfaces for network communication and allow multiple clients or servers to establish connections at the same time. The following are some common multi-socket applications:
Chat server: Chat server can establish connections with multiple clients at the same time, receive and send messages. Multiple sockets allow the server to handle multiple client connection requests at the same time, achieving efficient concurrent processing.
Game server: The game server needs to establish connections with multiple clients at the same time and handle game logic and data exchange. Multiple sockets allow the server to handle multiple client connection requests at the same time, improving the smoothness and stability of the game.
Live video server: The live video server needs to establish connections with multiple clients at the same time to transmit video data. Multiple sockets allow the server to handle multiple client connection requests at the same time, improving the smoothness and stability of live broadcasts.
Distributed system: A distributed system requires multiple servers to work together to process client requests. Multiple sockets allow each server to handle multiple client connection requests at the same time, improving the scalability and stability of the system.
3 WIZnet Ethernet chip
WIZnet mainstream hardware protocol stack Ethernet chip parameter comparison
Model | Embedded Core | Host I/F | TX/RX Buffer | HW Socket | Network Performance |
---|---|---|---|---|---|
W5100S | TCP/IPv4, MAC & PHY | 8bit BUS, SPI | 16KB | 4 | Max.25Mbps |
W6100 | TCP/IPv4/IPv6, MAC & PHY | 8bit BUS, Fast SPI | 32KB | 8 | Max.25Mbps |
W5500 | TCP/IPv4, MAC & PHY | Fast SPI | 32KB | 8 | Max 15Mbps |
W5100S/W6100 supports 8-bit data bus interface, and the network transmission speed will be better than W5500.
W6100 supports IPv6 and is compatible with W5100S hardware. If users who already use W5100S need to support IPv6, they can be Pin to Pin compatible.
W5500 has more Sockets and send and receive buffers than W5100S.
4 Overview and usage of multi-channel socket setting example
4.1 Flowchart
The running block diagram of the program is as follows:
4.2 Core preparation work
Software
Visual Studio Code
WIZnet UartTool
SocketTester
Hardware
W5100SIO module + RP2040 Raspberry Pi Pico development board or WIZnet W5100S-EVB-Pico development board
Micro USB interface data cable
TTL to USB
cable
4.3 Connection method
Connect the USB port of the PC through the data cable (mainly used for burning programs, but can also be used as a virtual serial port)
Convert TTL serial port to USB and connect the default pin of UART0:
RP2040 GPIO0 (UART0 TX) <----> USB_TTL_RX
RP2040 GPIO1 (UART0 RX) <----> USB_TTL_TX
When wiring using module connection RP2040
RP2040 GPIO16 <----> W5100S MISO
RP2040 GPIO17 <----> W5100S CS
RP2040 GPIO18 <----> W5100S SCK
RP2040 GPIO19 <----> W5100S MOSI
RP2040 GPIO20 <----> W5100S RST
Connect the PC and device to the router LAN port through network cables
4.4 Main code overview
We are using the official ioLibrary_Driver library of WIZnet. The library supports rich protocols and is easy to operate. The chip integrates the TCP/IP protocol stack on the hardware. The library also encapsulates the protocols above the TCP/IP layer. We only need to simply call the corresponding function to complete the application of the protocol. .
Step one: Add the corresponding .h file to the tcp_server_multi_socket.c file.
Step 2: Define the macros required for DHCP configuration.
Step 3: Configure network information and turn on DHCP mode.
Step 4: Write a timer callback processing function for the DHCP 1 second tick timer processing function.
Step 5: The main function first defines a timer structure parameter to trigger the timer callback function, initializes the serial port and SPI, then writes the network configuration parameters of W5100S, initializes DHCP and starts DHCP to obtain the IP. Print the obtained IP. When the number of acquisitions exceeds the maximum number of acquisitions, the static IP will be used, and the main loop will be executed in the server running function.
Step 6: We see the running function of the server function. First, open the socket, create the server, and then start monitoring. If a customer connects, the socket number will be incremented by one. W5100S supports up to 4 sockets, and W5500 supports up to 8 sockets. . Cyclically detect the messages sent by the client and print out the information corresponding to the connected client.
void do_tcp_server(void)
{
static uint8_t sock_cof = 0;
uint16_t len = 0;
uint8_t destip[4];
uint16_t destport;
switch (getSn_SR(sock_cof)) /*Get the socket status*/
{
case SOCK_CLOSED: /*The socket is closed*/
socket(sock_cof, Sn_MR_TCP, local_port, Sn_MR_ND); // Open socket
break;
case SOCK_INIT: /*The socket is in the initialization state*/
listen(sock_cof); /*socket starts listening*/
break;
case SOCK_ESTABLISHED: /*The socket is in the connection establishment state*/
if (getSn_IR(sock_cof) & Sn_IR_CON) /*Receive interrupt flags clearly*/
{
setSn_IR(sock_cof, Sn_IR_CON);
}
len = getSn_RX_RSR(sock_cof);
if (len > 0)
{
recv(sock_cof, buff, len); /*print the receive data.*/
buff[len] = 0x00;
getSn_DIPR(sock_cof, destip);
destport = getSn_DPORT(sock_cof);
printf("socket%d form:%d.%d.%d.%d port: %d message:%s\r\n", sock_cof, destip[0], destip[1], destip[2], destip[3], destport, buff);
send(sock_cof, buff, len);
}
break;
case SOCK_CLOSE_WAIT: /*The socket is waiting to be closed*/
close(sock_cof);
break;
}
if (sock_cof < 4)
{
sock_cof++;
}
else
{
sock_cof = 0;
}
if (sock_cof < 8)
{
sock_cof++;
}
else
{
sock_cof = 0;
}
}
4.5 Results demonstration
5 Precautions
If we want to use WIZnet's W5500 to implement the example in this chapter, we only need to modify two places:
(1) Find the wizchip_conf.h header file under library/ioLibrary_Driver/Ethernet/ and modify the WIZCHIP macro definition to W5500.
(2) Find the CMakeLists.txt file under library and set COMPILE_SEL to ON. OFF is W5100S and ON is W5500.