UDP Hamming code
Use Hamming code to improve UDP accuracy
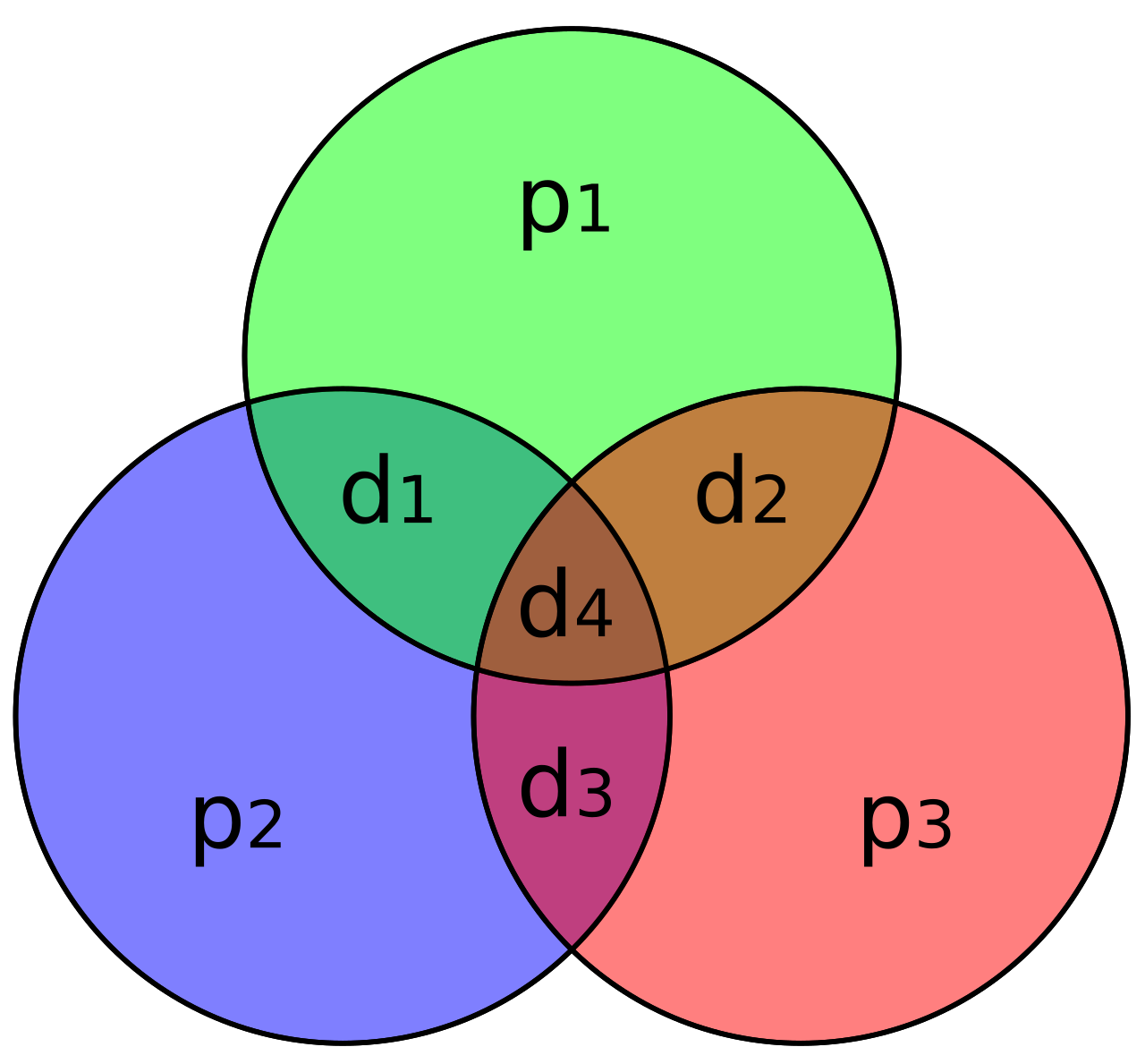
Story
Hamming code is a simple error correction code, which will be learned in the introduction to computer science in college. I want to try to use it to improve the accuracy of UDP transmission. But I was too lazy to write an encoding and decoding algorithm, so I used the lookup table method.
Coding
Table Generator
After writting and testing, running attchment file "hamming_code_7_4.ipynb". I got encode and decode table.
Encode table
[0, 15, 19, 28, 37, 42, 54, 57, 70, 73, 85, 90, 99, 108, 112, 127]
Decode table
[0, 0, 0, 2, 0, 4, 8, 1, 0, 9, 5, 1, 3, 1, 1, 1, 0, 2, 2, 2, 3, 10, 6, 2, 3, 7, 11, 2, 3, 3, 3, 1, 0, 4, 5, 12, 4, 4, 6, 4, 5, 7, 5, 5, 13, 4, 5, 1, 14, 7, 6, 2, 6, 4, 6, 6, 7, 7, 5, 7, 3, 7, 6, 15, 0, 9, 8, 12, 8, 10, 8, 8, 9, 9, 11, 9, 13, 9, 8, 1, 14, 10, 11, 2, 10, 10, 8, 10, 11, 9, 11, 11, 3, 10, 11, 15, 14, 12, 12, 12, 13, 4, 8, 12, 13, 9, 5, 12, 13, 13, 13, 15, 14, 14, 14, 12, 14, 10, 6, 15, 14, 7, 11, 15, 13, 15, 15, 15]
Encode before sending
Split one byte to two nibbles
def hamming_encode_table_method(plaintext):
return_list=list()
for s in range(len(plaintext)):
### for debug check
# print(s)
# codeword1 = encode_table[int(plaintext[s]/16)]
# print(codeword1)
return_list.append(encode_table[int(plaintext[s]/16)])
# codeword2 = encode_table[int(plaintext[s]%16)]
# print(codeword2)
return_list.append(encode_table[int(plaintext[s]%16)])
# print(return_bytes)
return bytes(return_list)
Decode
decode and combine two nibbles back to one byte
def hamming_decode_table_method(encryptext):
return_list=list()
for s in range(len(encryptext)):
if s % 2 ==0:
high_nibble=decode_table[int(encryptext[s])]
low_nibble=decode_table[int(encryptext[s+1])]
c_append=high_nibble*16+low_nibble
# print(high_nibble)
# print(low_nibble)
# print(c_append)
return_list.append(c_append)
return bytes(return_list)
running attachment main.py on W55RP20-EVB-PICO
and
running attachment UDP_hamming.ipynb (or UDP_hamming.py) on PC
Add random 1 bit error in each bytes in the transmitted data.
import random
def add_1bit_error(input_message):
for s in range(len(input_message)):
input_message[s] = (int(input_message[s]) ^ (1<< random.randint(0, 6)))
return input_message
I tested for 30 minutes and no second bit error occurred. Maybe it’s because the network line in my environment is very short.
-
Table generation Python code
Use for table generation, run on PC
-
UDP Hamming code micropython
run on W55RP20-EVB-PICO
-
UDP Hamming code python jupyternotebook
run on PC easy for debug
-
UDP Hamming code python
run on PC