Screen Time monitoring
Screen Time monitoring
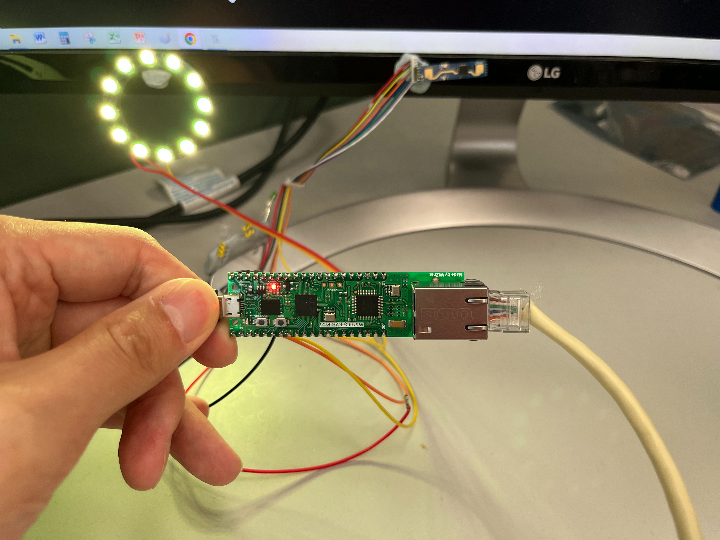
adafruit - Adafruit IO
x 1
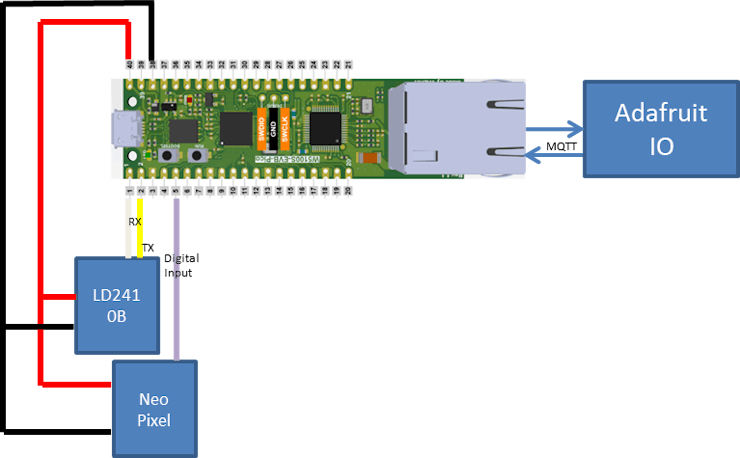
def collect_data (self, timeout: int = 5) -> None:
self.uart.reset_input_buffer()
gc.collect()
stamp = time.monotonic()
response = b""
while (time.monotonic() - stamp) < timeout:
if self.uart.in_waiting:
response += self.uart.read(1)
if Out_head in response and response[-4:] == Out_end:
break
#print(response)
#check data mode headers
self._check_head_tail("data",response)
if response[7] != int.from_bytes(Out_data_head,'big'):
raise ValueError ("Wrong Output data Header")
elif response[-6] != int.from_bytes(Out_data_end,'big'):
raise ValueError ("Wrong Output data End")
elif response[-5] != 0:
raise ValueError ("Wrong checking point")
#Checking operation Mode
if response[6] == 2:
self.W_type = "Basic Mode"
elif response[6] == 1:
self.W_type = "Engineering Mode"
else:
raise ValueError ("Wrong Working mode Data")
#Checking target:
if response[8] == 0:
self.target = "No target"
elif response[8] == 1:
self.target = "Moving target"
elif response[8] == 2:
self.target = "Stable target"
elif response[8] == 3:
self.target = "Both target"
else:
raise ValueError ("Wrong Rarget Value")
#Convert all the data
self.move_dist = response[10]*256 + response[9]
self.move_sen = response[11]
self.stable_dist = response[13]*256 + response[12]
self.stable_sen = response[14]
self.M_dist = response[16]*256 + response[15]
@property
def cmd_mode(self): #check the command mode is it on / off
return self.cmd_check # 0 = OFF , 1 = ON
@cmd_mode.setter #set the command mode
def cmd_mode(self, value):
#Set the codes into correct format before send
if value == 1:
data = Command_head + self._shifting(4) +Command_mode_on + Command_end
elif value == 0:
data = Command_head + self._shifting(2) +Command_mode_off + Command_end
result =self._send_command(data) #send the data to the module
#Received feedback - Print out the result and change the command mode staus
if result[0] == Command_mode_on[0]:
print("Command Mode ON")
self.cmd_check = 1
elif result[0] == Command_mode_off[0]:
print("Command Mode OFF")
self.cmd_check = 0
def set_sensitivity(self,unit_sen: str, move_sen: int, stable_sen: int):
padding = b"\x00\x00"
move = b"\x01\x00"
stable = b"\x02\x00"
try:
unit = self._shifting(int(unit_sen))
except ValueError as error:
if unit_sen is "all":
unit = b"\xFF\xFF"
else:
raise ValueError ("Wrong sensitivity Input")
except Exception as error:
raise error
data = Command_head + self._shifting(20) + sensitivity + padding + unit + padding
data = data + move + self._shifting(move_sen) + padding
data = data + stable + self._shifting(stable_sen) + padding + Command_end
result =self._send_command(data)
print ("Sensitivity has been Set!")
def _send_command (self,cmd: str, timeout: int = 5) -> None:
#print(cmd)
self.uart.write(cmd)
self.uart.reset_input_buffer()
gc.collect()
stamp = time.monotonic()
response = b""
while (time.monotonic() - stamp) < timeout:
if self.uart.in_waiting:
response += self.uart.read(1)
if Command_head in response and response[-4:] == Command_end:
break
#print(response)
#print(type(response))
self._check_head_tail("command",response)
if response[6] + response[7] != cmd[6] + cmd[7] + 1:
raise ValueError("Command Word Response Error")
elif response[8] + response[9] != 0:
raise ValueError("Command Fail")
return response[6:-4]
def _check_head_tail(self,mode, response) -> None:
if mode is "data":
head = Out_head
tail = Out_end
output = "Output"
elif mode is "command":
head = Command_head
tail = Command_end
output = "Command"
else:
raise ValueError ("Wrong head tail mode Input")
if response[0:4] != head:
raise ValueError ("Wrong {} Header".format(output))
elif response[-4:] != tail:
raise ValueError ("Wrong {} End".format(output))
elif response[5]*256 + response[4] != len(response) - len(Out_head) - len(Out_end) - 2:
raise ValueError ("Missing {} data".format(output))
#function to collect the screen time from adafruit IO
def waiting_time(client, topic, message):
global time_recorder
# Method callled when a client's subscribed feed has a new value.
print("New message 2 on topic {0}: {1}".format(topic, message))
time_recorder = int(message)
#function to collect reset response from adafruit IO
def reset(client, topic, message):
# Method callled when a client's subscribed feed has a new value.
print("New message on topic {0}: {1}".format(topic, message))
if message == "0":
flag.value = 1
#Main function to manage the screen time operation
def scan(time_value,temp,counter,alert_counter,leave, led):
global time_recorder
#collect data function from LD2410B module library
dist_sen.collect_data()
#check the sensor's targetting object
if dist_sen.target == "Both target":
if dist_sen.move_dist >= dist_sen.stable_dist: #compare the distance - distance value is more accurate
data = dist_sen.move_dist
else:
data = dist_sen.stable_dist
elif dist_sen.target == "Moving target": #If it detects the moving object, choose moving target
data = dist_sen.move_dist
elif dist_sen.target == "Stable target": #Since the distance between the module is short, stable target could be consider to use.
data = dist_sen.stable_dist
if data == 8: #if it shows 8 value, it means error.
data = dist_sen.move_dist
else:
data = None # No target, just ignore
if data != None
# the range that I will be seated - If detected
if data > 65 and data < 100:
leave = False # Turn off leave flag
temp = time.time() #collect current time
if time_value is None:
time_value = temp # Starting Point
else: #check current status for LED display
difference = temp - time_value
if difference <= (time_recorder /3): #Beginning period with the screen
print ("Green Light")
pixels.fill((38,252,5)) # Display green light
pixels.show()
led += 1
if led == 1: #Upload once to adafruit IO
io.publish("light", "#26fc05") #Upload green light status
elif difference > (time_recorder /3) and difference < time_recorder: #it has been for a while with the screen
print ("Yellow Light")
if led >= 1:
led = 0
pixels.fill((250,242,7)) # Display Yellow Light
pixels.show()
led -= 1
if led == -1: #Upload once to adafruit IO
io.publish("light", "#faf207")
elif difference >= time_recorder: #If it has passed or equal to the waiting time
print("Over time! - Red Light")
alert_counter += 1
if alert_counter == 1: #Activate the alert section
io.publish("alert", "OverTime") #posted to adafruit IO to show it has passed the screening time
io.publish("light", "#fc0905") #showed red on adafruit IO
pixels.fill((252,9,5)) # sDisplay Red Light
pixels.show()
else: #detected No one is in front of the screen
if leave is False: #confirmed the previous moments are present in front of the screen
counter += 1
if temp is not None: #if the previous moment is present in front of the screen
counter = 0 #reset counter
temp = None
print (counter)
if counter > 3: #if accumulated for 3 times, confirmed no one is in front of the screen.
#reset everything
temp = time.time()
difference = temp - time_value
print ("You have looked on the screen for {} seconds".format(difference))
counter = 0
time_value = None
temp = None
alert_counter = 0
leave = True
led = 0
pixels.fill((0,0,0)) # set to turn off the pixel
pixels.show() #present to off
io.publish("light", "#000000")
return time_value, temp, counter, alert_counter,leave,led
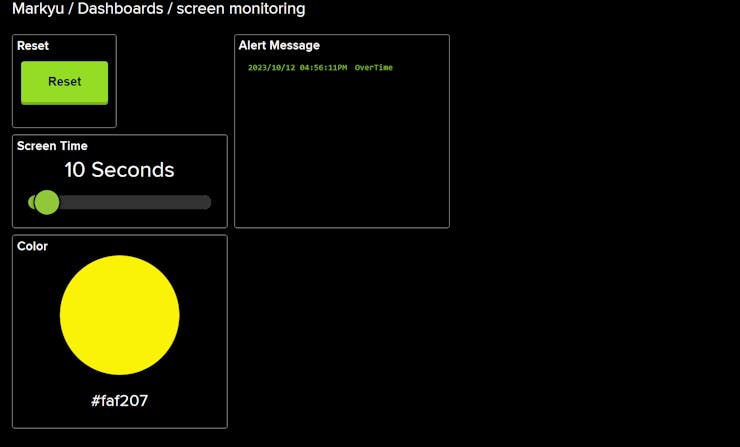
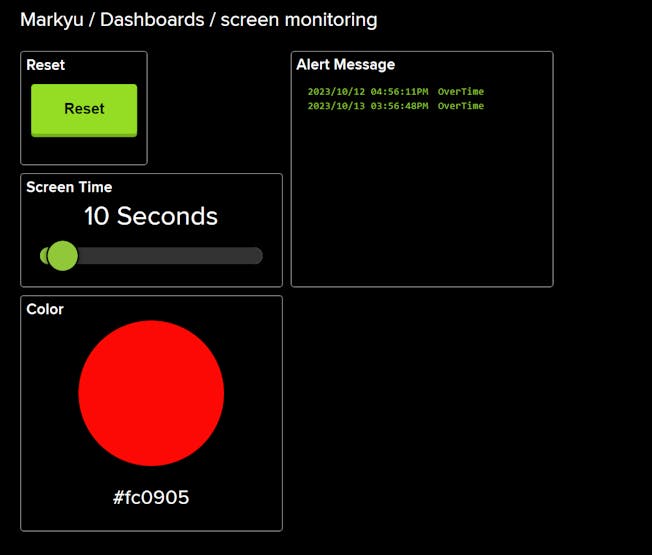