W55RP20 MicroPython Examples for tcp client
This MicroPython project establishes a TCP client using a WIZnet W5x00 Ethernet module, continuously connecting to a server and echoing received data.
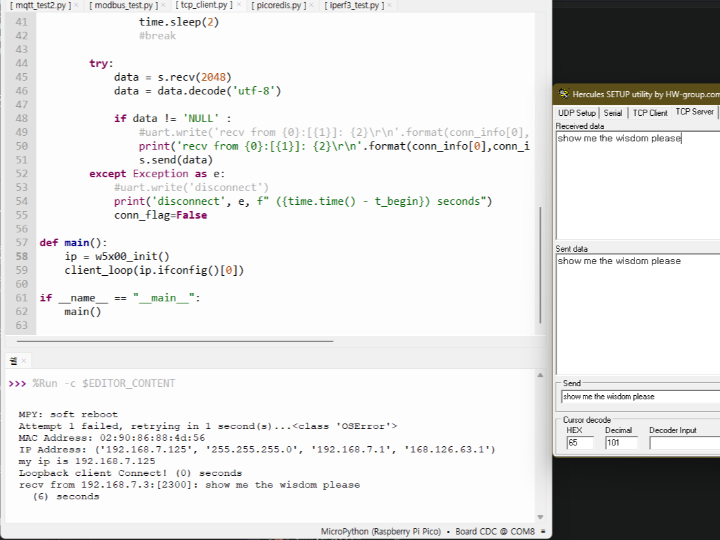
Source Code Explanation
Imports:
socket
,usocket
: Used for TCP communication.machine
,network
: Handles hardware and networking functionalities.time
,uasyncio
: Provides timing and async support.w5x00_init
: Initializes the WIZnet W5x00 Ethernet module.
Connection Settings:
destip = '192.168.7.3'
anddestport = 2300
define the target server.conn_info = (destip, destport)
holds the connection tuple.conn_flag
: Tracks connection status.
client_loop(ip)
:
- Establishes a persistent TCP connection to the server.
- Continuously attempts reconnection if the connection fails.
- Receives data (
s.recv(2048)
), decodes it, prints it, and echoes it back.
main()
:
- Calls
w5x00_init()
to configure the W5x00 Ethernet module. - Extracts IP and starts
client_loop(ip)
.
Execution Flow:
- Runs as
__main__
, initializes Ethernet, and starts the TCP client.
▶ How to Run
Prepare Hardware:
- Connect the WIZnet W5x00 Ethernet module to the MicroPython board.
- Ensure the board is networked via Ethernet.
Deploy Code:
- Upload the script to the MicroPython board.
- Run
main.py
via REPL or automatically on boot.
Set Up a TCP Server (for testing):
nc -lvp 2300
Or use a Python TCP server:
import socket
s = socket.socket()
s.bind(('0.0.0.0', 2300))
s.listen(1)
conn, addr = s.accept()
while True:
data = conn.recv(2048)
if data:
conn.send(data)
Execution Result
- If connection succeeds:
my ip is 192.168.7.100
Loopback client Connect! (0.5) seconds
recv from 192.168.7.3:[2300]: Hello Server
- If the server disconnects:
disconnect [Errno 104] ECONNRESET
connect error [Errno 111] ECONNREFUSED
- Data Echoing:
- Any received message is echoed back to the sender.