TCP/IP Client server with STM32
This project demonstrates how to establish reliable and efficient Ethernet communication using the ESP32 microcontroller and the W5500 Ethernet module.
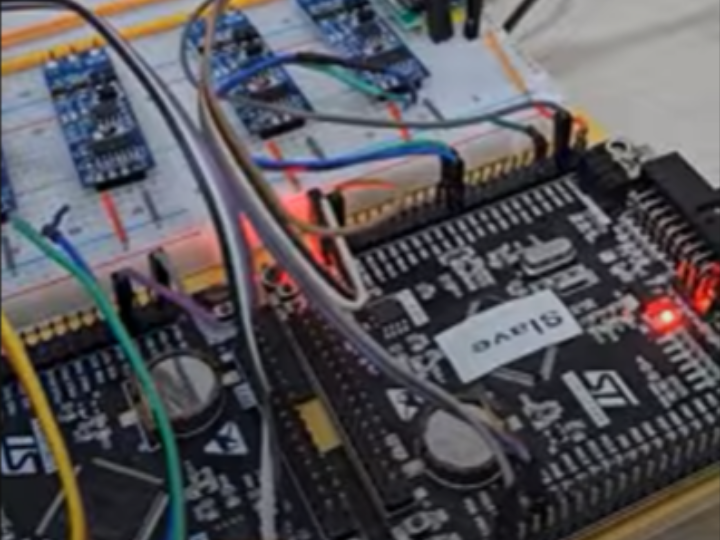
1. Project Overview & Features
Core Functionality:
- Implements stable Ethernet communication using an ESP32 microcontroller and a W5500 Ethernet module.
Problem Solved & Purpose:
- Resolves wireless connectivity issues by providing a more stable wired network for IoT applications.
- Improves network reliability and speed for devices requiring a consistent connection.
Key Features:
- Hardware: ESP32 microcontroller, W5500 Ethernet module
- Network: Wired Ethernet via W5500
- Protocol: TCP/IP socket communication
- Data Handling: Utilizes ESP32 for data transmission
2. Hardware & Software Stack
Hardware:
- MCU: ESP32 board
- Network Module: W5500 Ethernet module
- Additional Peripherals: No specific sensor mentioned, but can integrate with various sensors
Software:
- Programming Language: C/C++
- Libraries Used:
- Arduino Ethernet library
- SPI library
- Network Protocol: TCP/IP
3. Key Parts of the Source Code
Ethernet Initialization & Setup
#include <Ethernet.h>
#include <SPI.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress ip(192, 168, 1, 177);
void setup() {
Ethernet.begin(mac, ip);
// Additional setup code
}
Server Socket Creation & Client Handling
EthernetServer server(80);
void loop() {
EthernetClient client = server.available();
if (client) {
// Process client requests
}
}
4. Comparison with Traditional Implementations
Advantages:
✅ Stable Wired Connection: More reliable than Wi-Fi, with fewer interference issues.
✅ Hardware-Based TCP/IP Offloading: The W5500 module handles networking independently, reducing the ESP32’s processing load.
Disadvantages:
❌ Limited Installation Flexibility: Wired connections restrict placement options.
❌ Additional Hardware Cost: Adding a W5500 module increases cost and circuit complexity.
5. Suggested Improvements & Enhancements
✔ Security Enhancements: Implement TLS/SSL encryption for secure data transmission.
✔ Error Handling: Add mechanisms to detect and handle network failures and unexpected errors.
✔ Data Logging: Enable local storage (SD card) or cloud database integration for long-term data tracking.
✔ Real-time Monitoring: Implement a web server interface or integrate with Node-RED for real-time data visualization.