RE> W5500_TCP_Server
The blog post on CSDN provides a comprehensive guide on integrating STM32 microcontrollers with the W5500 Ethernet controller. This integration enables seamless
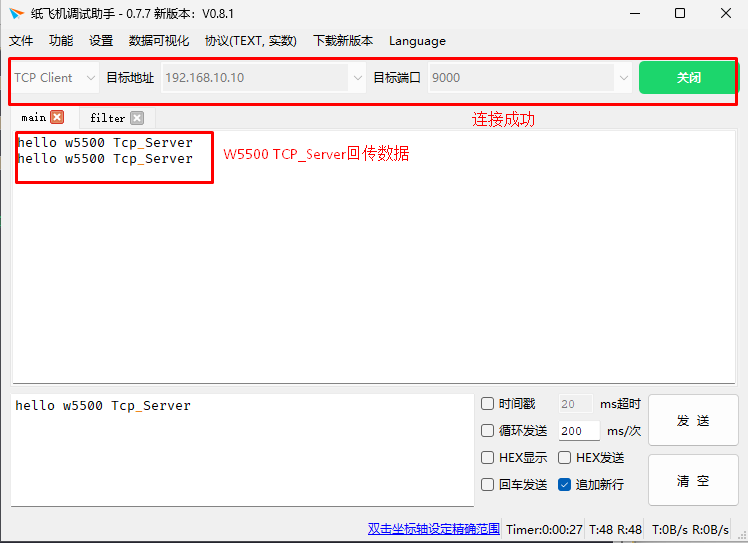
Comprehensive Guide to STM32 Ethernet Integration with W5500
Overview
This guide provides detailed instructions for integrating STM32 microcontrollers with the W5500 Ethernet controller, including hardware connections, software configuration, and example code to facilitate network communication.
Hardware Setup
- Connections:
- Connect the W5500 module to the STM32 microcontroller using SPI pins.
- Ensure connections are made as per the specified pin layout in the guide.
Software Configuration
Library Integration:
- Download the necessary libraries from the provided sources.
- Integrate the libraries into your STM32 project.
Network Initialization:
- Configure network settings in the code.
- Initialize the W5500 module using provided functions.
Example Code
Below is an example of initializing the W5500 and setting up the network:
c
#include "w5500.h"
#include "stm32f1xx_hal.h"
// Define SPI handle
SPI_HandleTypeDef hspi1;
// Function to initialize W5500
void W5500_Init(void) {
// Initialize SPI
MX_SPI1_Init();
// Reset W5500
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_0, GPIO_PIN_RESET);
HAL_Delay(1);
HAL_GPIO_WritePin(GPIOB, GPIO_PIN_0, GPIO_PIN_SET);
HAL_Delay(200);
// Configure network settings
uint8_t mac[6] = {0x00, 0x08, 0xDC, 0x01, 0x02, 0x03};
uint8_t ip[4] = {192, 168, 0, 100};
uint8_t gateway[4] = {192, 168, 0, 1};
uint8_t subnet[4] = {255, 255, 255, 0};
wizchip_init(mac, ip, gateway, subnet);
// Check PHY status
if (getPHYStatus() == PHY_LINK_ON) {
printf("Ethernet cable connected.\n");
} else {
printf("Ethernet cable not connected.\n");
}
}
int main(void) {
HAL_Init();
SystemClock_Config();
MX_GPIO_Init();
MX_SPI1_Init();
// Initialize W5500
W5500_Init();
while (1) {
// Main loop
}
}
Conclusion
Integrating STM32 with the W5500 Ethernet controller is straightforward with the right guidance. This tutorial covers the essential steps for both hardware and software setup, providing a strong foundation for adding network capabilities to embedded projects.
For more detailed information, visit the original blog post on CSDN.