Use ChatGPT to develop W7500S2E-D1 Hardware test Code which Deployed in the W5100S_EVB_PICO
Use ChatGPT to develop W7500S2E-D1 Hardware test Code which Deployed in the W5100S_EVB_PICO
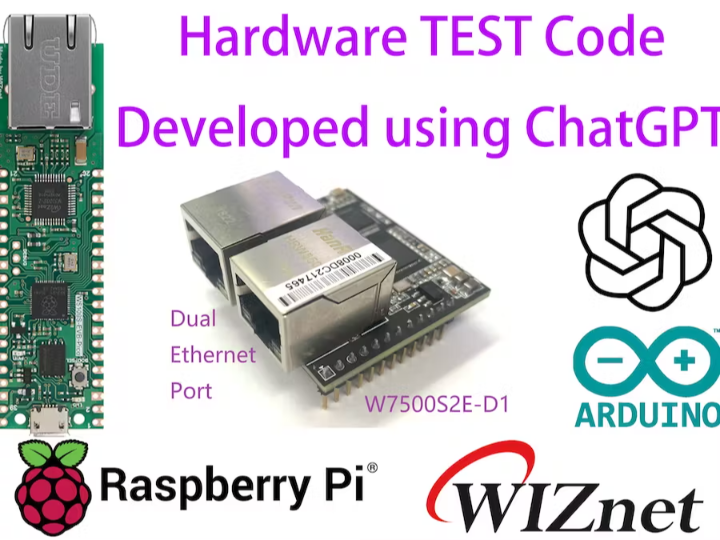

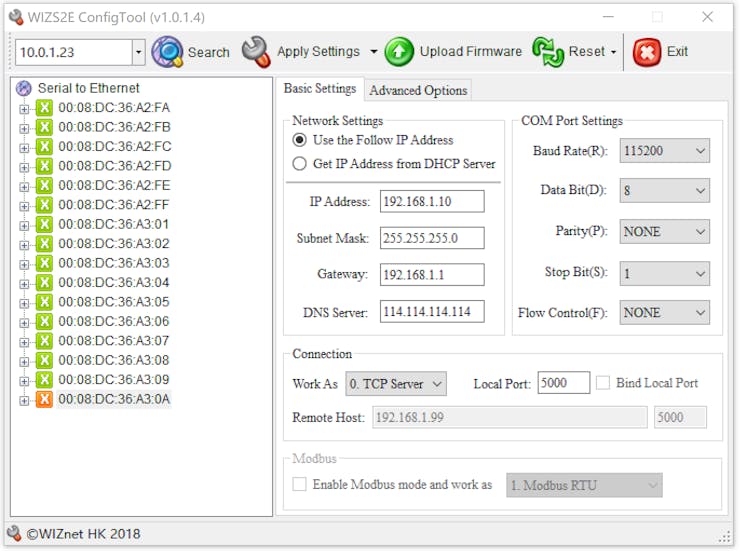

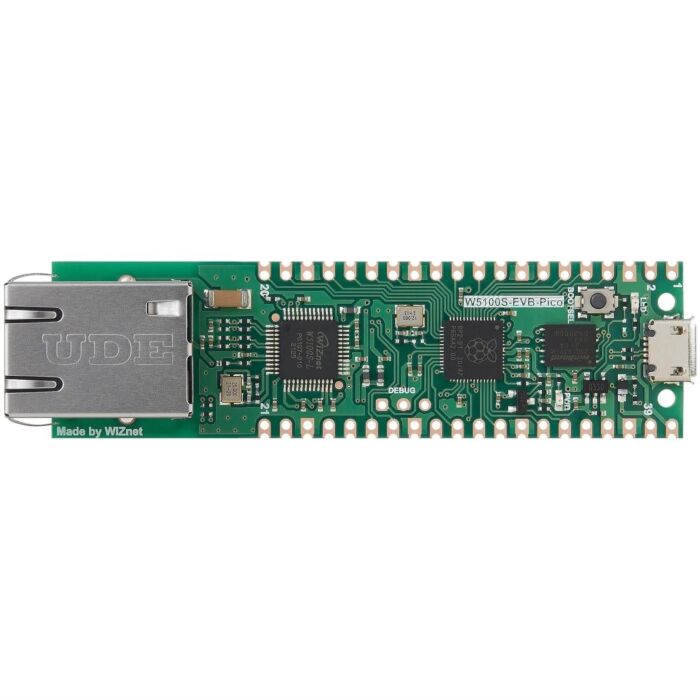
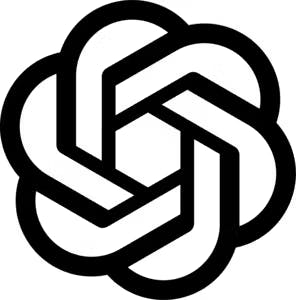
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress ip(192, 168, 0, 100);
IPAddress subnet(255, 255, 255, 0);
EthernetServer server(80); // Initialize Ethernet server on port 80
void setup() {
Ethernet.begin(mac, ip, subnet);
server.begin();
}
#include <EthernetUdp.h>
UDP udp;
void setup() {
udp.begin(8888);
}
void sendBroadcastPacket() {
IPAddress broadcastIp(255, 255, 255, 255); // Broadcast address
uint16_t port = 1234; // Broadcast port
udp.beginPacket(broadcastIp, port);
udp.write("Hello, world!"); // Write your data to the packet
udp.endPacket();
}
#include <SPI.h>
#include <Ethernet.h>
#include <EthernetUdp.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress ip(192, 168, 0, 100);
IPAddress subnet(255, 255, 255, 0);
EthernetUDP udp;
void setup() {
Ethernet.begin(mac, ip, subnet);
udp.begin(8888);
Serial.begin(9600);
}
void loop() {
sendBroadcastPacket();
delay(1000);
}
void sendBroadcastPacket() {
IPAddress broadcastIp(255, 255, 255, 255); // Broadcast address
uint16_t port = 1234; // Broadcast port
udp.beginPacket(broadcastIp, port);
udp.write("Hello, world!"); // Write your data to the packet
udp.endPacket();
Serial.println("Broadcast packet sent.");
}
#include <SPI.h>
#include <Ethernet.h>
#include <EthernetUdp.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress ip(192, 168, 0, 100);
IPAddress subnet(255, 255, 255, 0);
EthernetUDP udp;
void setup() {
Ethernet.begin(mac, ip, subnet);
udp.begin(8888);
Serial.begin(9600);
}
void loop() {
sendBroadcastPacket();
receivePackets();
delay(1000);
}
void sendBroadcastPacket() {
IPAddress broadcastIp(255, 255, 255, 255); // Broadcast address
uint16_t port = 1234; // Broadcast port
udp.beginPacket(broadcastIp, port);
udp.write("Hello, world!"); // Write your data to the packet
udp.endPacket();
Serial.println("Broadcast packet sent.");
}
void receivePackets() {
int packetSize = udp.parsePacket();
if (packetSize) {
char packetData[255];
int len = udp.read(packetData, sizeof(packetData) - 1);
if (len > 0) {
packetData[len] = '\0';
Serial.print("Received packet: ");
Serial.println(packetData);
}
}
}
#include <SPI.h>
#include <Ethernet.h>
#include <EthernetUdp.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress ip(192, 168, 0, 100);
IPAddress subnet(255, 255, 255, 0);
EthernetUDP udp;
void setup() {
Ethernet.begin(mac, ip, subnet);
udp.begin(8888);
Serial.begin(9600);
}
void loop() {
sendBroadcastPacket();
delay(1000);
printReceivedPackets();
}
void sendBroadcastPacket() {
IPAddress broadcastIp(255, 255, 255, 255); // Broadcast address
uint16_t port = 1234; // Broadcast port
udp.beginPacket(broadcastIp, port);
udp.write("Hello, world!"); // Write your data to the packet
udp.endPacket();
Serial.println("Broadcast packet sent.");
}
void printReceivedPackets() {
unsigned long startTime = millis();
while (millis() - startTime < 1000) {
int packetSize = udp.parsePacket();
if (packetSize) {
char packetData[255];
int len = udp.read(packetData, sizeof(packetData) - 1);
if (len > 0) {
packetData[len] = '\0';
Serial.print("Received packet: ");
Serial.println(packetData);
}
}
}
}
#include <SPI.h>
#include <Ethernet.h>
#include <EthernetUdp.h>
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
IPAddress ip(192, 168, 0, 100);
IPAddress subnet(255, 255, 255, 0);
EthernetUDP udp;
struct DataPacket {
IPAddress senderIP;
uint16_t senderPort;
char data[255];
};
DataPacket receivedPacket;
void setup() {
Ethernet.begin(mac, ip, subnet);
udp.begin(8888);
Serial.begin(9600);
}
void loop() {
sendBroadcastPacket();
delay(1000);
printReceivedPackets();
}
void sendBroadcastPacket() {
IPAddress broadcastIp(255, 255, 255, 255); // Broadcast address
uint16_t port = 1234; // Broadcast port
udp.beginPacket(broadcastIp, port);
udp.write("Hello, world!"); // Write your data to the packet
udp.endPacket();
Serial.println("Broadcast packet sent.");
}
void printReceivedPackets() {
unsigned long startTime = millis();
while (millis() - startTime < 1000) {
int packetSize = udp.parsePacket();
if (packetSize) {
udp.read((byte*)&receivedPacket, sizeof(receivedPacket));
Serial.println("Received packet:");
Serial.print(" Sender IP: ");
Serial.println(receivedPacket.senderIP);
Serial.print(" Sender Port: ");
Serial.println(receivedPacket.senderPort);
Serial.print(" Data: ");
Serial.println(receivedPacket.data);
}
}
}
#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0x00, 0x08, 0xdc, 0x11, 0x22, 0x33 }; // Your MAC Address
IPAddress localIP(192, 168, 1, 254); // Your IP Address
IPAddress broadcastIp(255, 255, 255, 255); // Broadcast address
EthernetUDP udp;
EthernetServer server(80);
uint8_t count = 0;
unsigned long startTime;
#pragma pack(1)
struct S2E_DATA {
char SERH[4];
uint8_t MAC[6];
uint8_t DEVICE_ID[16];
uint8_t STATE;
uint8_t SW_VER[2];
char DEVICE_TYPE[16];
char DEVICE_NAME[16];
char DEVICE_PWD[16];
uint16_t HTTP_PORT;
uint32_t FW_LEN;
uint32_t FW_CHECKSUM;
uint8_t DEBUG_FLAG;
uint8_t ECHO_FLAG;
uint8_t NETBIOS;
uint8_t DHCP;
uint8_t LOCAL_IP[4];
uint8_t SUBNET[4];
uint8_t GATEWAY[4];
uint8_t DNS[4];
uint8_t BAUDRATE;
uint8_t DATABIT;
uint8_t PARITY;
uint8_t STOPBIT;
uint8_t FLOWCONTROL;
uint8_t MODE;
uint16_t LOCAL_PORT;
uint8_t REMOTE_IP[4];
uint16_t REMOTE_PORT;
uint8_t DNS_FLAG;
char DOMAIN[32];
uint16_t INACTIVITY;
uint16_t RECONNECT;
uint16_t NAGLE_TIME;
uint16_t PACK_LEN;
uint16_t KEEP_ALIVE;
uint8_t BIND_PORT;
uint8_t LINK_CLEAR_BUF;
uint8_t LINK_PWD;
uint8_t LINK_MSG;
uint8_t LINK_CONDITION;
uint8_t START_MODE;
uint16_t CRC16;
uint32_t NET_SEND_NUM;
uint32_t NET_RCV_NUM;
uint32_t SEC_SEND_NUM;
uint32_t SEC_RCV_NUM;
uint32_t ON_TIME;
uint8_t RUN_MODE;
uint8_t TEMPERATURE;
};
S2E_DATA s2eData;
S2E_DATA s2eDataArray[16];
void setup() {
Serial.begin(115200);
while (!Serial) {
delay(100);
}
Ethernet.init(17); // WIZnet W5100S-EVB-Pico
Ethernet.begin(mac, localIP);
// start the server
server.begin();
Serial.print("server is at ");
Serial.println(Ethernet.localIP());
udp.begin(8888);
Serial.println("Broadcast test");
}
void loop() {
count = 0;
sendBroadcastPacket();
printReceivedPackets();
startTime = millis();
while (millis() - startTime < 2000) {
}
}
void sendBroadcastPacket() {
char data[] = "SERH"; // Discovery String
udp.beginPacket(broadcastIp, 1460); // open udp socket
udp.write(data); // Write your data to the packet
udp.endPacket(); // end
Serial.println("Discovery packet sent.");
}
void printReceivedPackets() {
startTime = millis();
while (millis() - startTime < 500) {
int packetSize = udp.parsePacket();
if (packetSize) {
int len = udp.read((byte*)&s2eData, sizeof(s2eData) - 1);
if (len > 0) {
for(uint8_t m=0; m<16; m++)
{
if((s2eDataArray[m].MAC[3] != s2eData.MAC[3])||(s2eDataArray[m].MAC[4] != s2eData.MAC[4])||(s2eDataArray[m].MAC[5] != s2eData.MAC[5]))
{
s2eDataArray[m] = s2eData;
count ++;
Serial.print("Module NO.");
Serial.print(count);
Serial.print(" ");
Serial.print(s2eDataArray[m].DEVICE_TYPE);
Serial.print(" ");
Serial.print("MAC:");
for (int i = 0; i < 6; i++) {
Serial.printf("%02X",s2eDataArray[m].MAC[i]);
Serial.print(' ');
}
Serial.print("IP:");
for (int n = 0; n < 4; n++) {
Serial.print(s2eDataArray[m].LOCAL_IP[n]);
if(n<3)
{
Serial.print('.');
}
else
{
break;
}
}
Serial.println();
break;
}
}
}
}
}
}
//#define ETHERNET_LARGE_BUFFERS
#define ETHERNET_LARGE_BUFFERS
#define MAX_SOCK_NUM 8
#define MAX_SOCK_NUM 2

#include <SPI.h>
#include <Ethernet.h>
byte mac[] = { 0x00, 0x08, 0xdc, 0x11, 0x22, 0x33 }; // Your MAC Address
IPAddress localIP(192, 168, 1, 254); // Your IP Address
EthernetServer server(80);
struct S2E_DATA {
// Your structure members here
};
S2E_DATA s2eDataArray[16];
void setup() {
Serial.begin(115200);
while (!Serial) {
delay(100);
}
Ethernet.init(17); // WIZnet W5100S-EVB-Pico
Ethernet.begin(mac, localIP);
server.begin();
Serial.print("Server is running at ");
Serial.println(Ethernet.localIP());
}
void loop() {
EthernetClient client = server.available();
if (client) {
processRequest(client);
client.stop();
}
}
void processRequest(EthernetClient client) {
String request = client.readStringUntil('\r');
client.readStringUntil('\n');
if (request.indexOf("GET /") != -1) {
sendResponse(client);
}
}
void sendResponse(EthernetClient client) {
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE html>");
client.println("<html>");
client.println("<head>");
client.println("<title>S2E Data</title>");
client.println("</head>");
client.println("<body>");
client.println("<h1>S2E Data</h1>");
for (int i = 0; i < 16; i++) {
client.print("<h2>Module NO.");
client.print(i + 1);
client.print("</h2>");
client.print("<p>DEVICE TYPE: ");
client.print(s2eDataArray[i].DEVICE_TYPE);
client.print("</p>");
client.print("<p>MAC: ");
for (int j = 0; j < 6; j++) {
client.print(String(s2eDataArray[i].MAC[j], HEX));
if (j < 5) {
client.print(":");
}
}
client.print("</p>");
client.print("<p>IP: ");
client.print(s2eDataArray[i].LOCAL_IP[0]);
client.print(".");
client.print(s2eDataArray[i].LOCAL_IP[1]);
client.print(".");
client.print(s2eDataArray[i].LOCAL_IP[2]);
client.print(".");
client.print(s2eDataArray[i].LOCAL_IP[3]);
client.print("</p>");
client.println("<hr>");
}
client.println("</body>");
client.println("</html>");
}
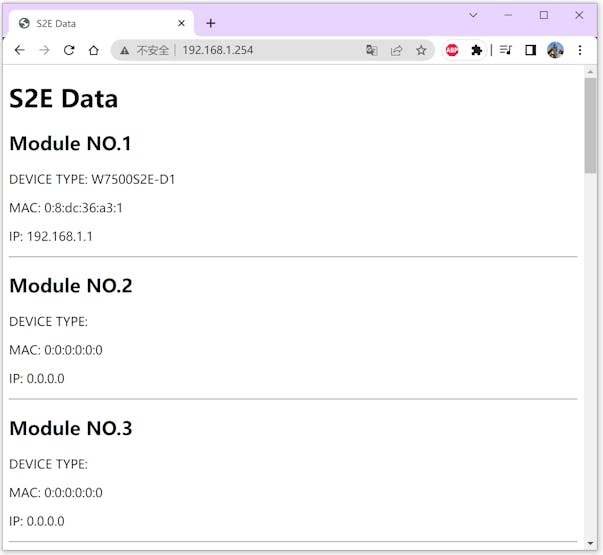
void sendResponse(EthernetClient client) {
client.println("HTTP/1.1 200 OK");
client.println("Content-Type: text/html");
client.println("Connection: close");
client.println();
client.println("<!DOCTYPE html>");
client.println("<html>");
client.println("<head>");
client.println("<title>WIZS2E ConfigTool Webpage</title>");
client.println("<meta http-equiv=\"refresh\" content=\"5\">");
client.println("<style>");
client.println(".device-type { color: green; font-size: 14px; }");
client.println("</style>");
client.println("</head>");
client.println("<body>");
client.println("<h1>WIZS2E ConfigTool Webpage</h1>");
for (int i = 0; i < 16; i++) {
if (s2eDataArray[i].DEVICE_TYPE[0] == '\0') {
continue; //skip invalid data
}
client.print("<h2>Module NO.");
client.print(i + 1);
client.print("</h2>");
client.print("<p><span class=\"device-type\">DEVICE TYPE: ");
client.print(s2eDataArray[i].DEVICE_TYPE);
client.print("</span></p>");
client.print("<p>MAC: ");
for (int j = 0; j < 6; j++) {
client.print(String(s2eDataArray[i].MAC[j], HEX));
if (j < 5) {
client.print(":");
}
}
client.print("</p>");
client.print("<p>IP: ");
client.print(s2eDataArray[i].LOCAL_IP[0]);
client.print(".");
client.print(s2eDataArray[i].LOCAL_IP[1]);
client.print(".");
client.print(s2eDataArray[i].LOCAL_IP[2]);
client.print(".");
client.print(s2eDataArray[i].LOCAL_IP[3]);
client.print("</p>");
client.println("<hr>");
}
client.println("</body>");
client.println("</html>");
}

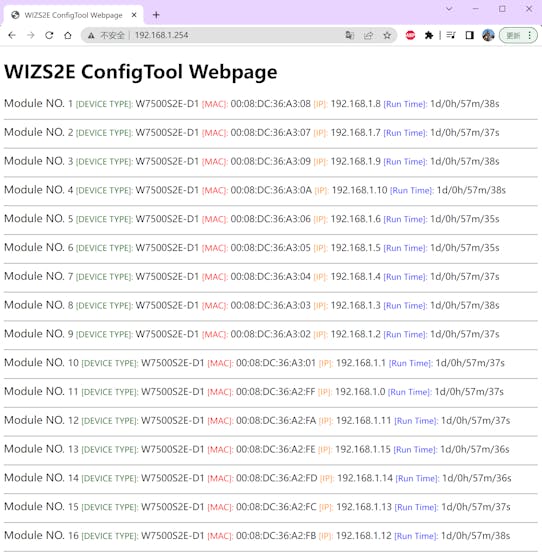
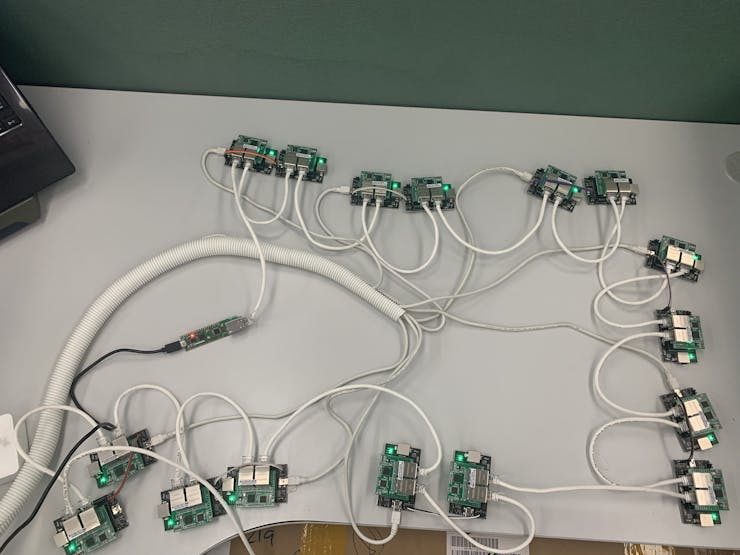
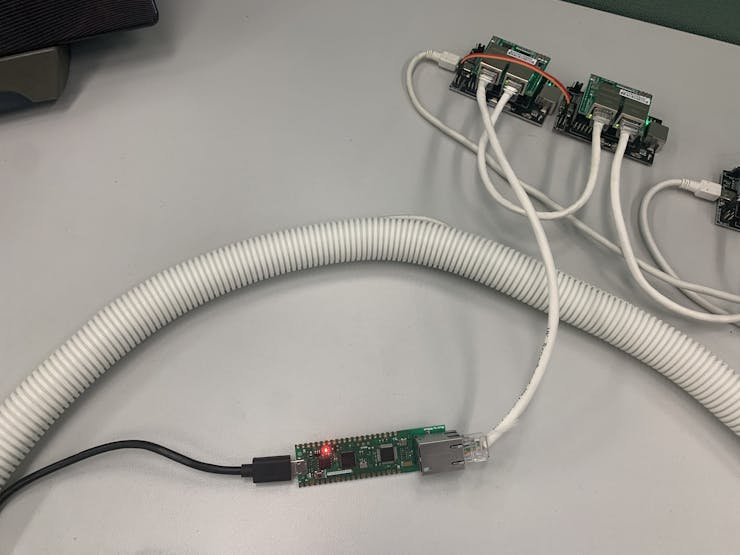
-
Hardware test Code for W7500S2E-D1
Hardware test Code for W7500S2E-D1
-
Hardware test Code for W7500S2E-D1
Github