Recommended Weather-Appropriate Song Playlists by using W5x00-EVB-Pico with DHT11 & MQTT protocol
Created a project using a W5100S-EVB-PICO board with a DHT11 sensor to collect weather data. Utilized MQTT communication to recommend playlists based on weather
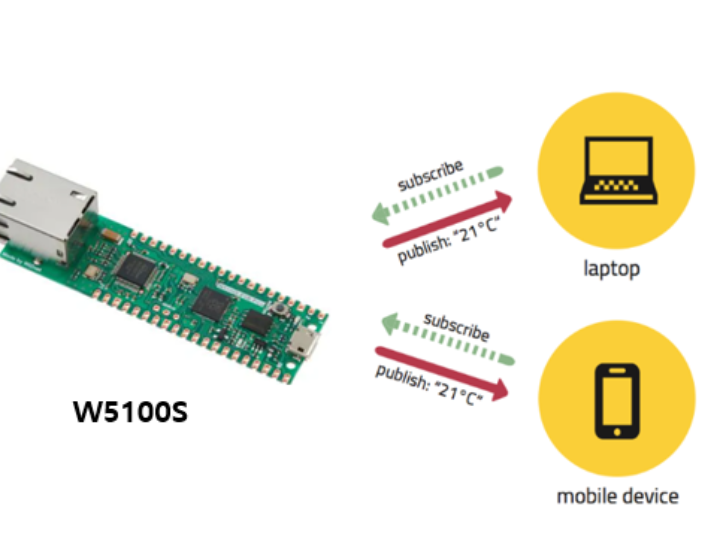
Software Apps and online services
I have created an application that receives temperature and humidity values from DHT11 via W5100S and recommends music appropriate for these values.
For this project, we collaborated with Hannah from Wiznet. She was responsible for MQTT communication control, while I focused on controlling the DHT11 and processing the data. I will explain the technology related to DHT11 control and data processing.
To reference the project files, please click below.
To start this project, connect the DHT11 and W5100S. Note that the DATA PIN number for DHT11 is 28. Also, connect the remaining 3.3V voltage and ground.
First, configure your device appropriately at the top of the entire code. This project is not limited to DHT11 and can also be used with other versions of DHT by making code modifications. If you want to use different pin numbers, please modify the port numbers.
// change this to match your setup
static const dht_model_t DHT_MODEL = DHT22;
static const uint DATA_PIN = 28;
static float celsius_to_fahrenheit(float temperature) {
return temperature * (9.0f / 5) + 32;
We have divided the sections into three categories: hot, cold, and warm. Using temperature-based conditional statements with the data from the DHT11 sensor at the bottom, we can customize the song recommendation data output differently.
stdio_init_all();
char dht_string[600];
char today_hot[500] = "Today's weather is Hot Summer! \nRecommended playlist: https://www.youtube.com/embed/Hf4A6O6q16c";
char today_cold[500] = "Today's weather is Cold! \nRecommended playlist: https://www.youtube.com/embed/K81dHzC0T_M";
char today_warm[500] = "Today's weather is Warm day! \nRecommended playlist: https://www.youtube.com/embed/DpcJP-wQWGI";
dht_t dht;
dht_init(&dht, DHT_MODEL, pio0, DATA_PIN, true /* pull_up */);
dht_start_measurement(&dht);
float humidity;
float temperature_c;
dht_result_t result = dht_finish_measurement_blocking(&dht, &humidity, &temperature_c);
if (result == DHT_RESULT_OK)
{
snprintf(dht_string, sizeof(dht_string), "%.1f C (%.1f F), %.1f%% humidity\n",
temperature_c, celsius_to_fahrenheit(temperature_c), humidity);
if(temperature_c > 30) strcat(dht_string, today_hot);
else if (temperature_c < 10) strcat(dht_string, today_cold);
else strcat(dht_string, today_warm);
}
else if (result == DHT_RESULT_TIMEOUT)
{
puts("DHT sensor not responding. Please check your wiring.");
}
else
{
assert(result == DHT_RESULT_BAD_CHECKSUM);
puts("Bad checksum");
}
The communication mechanism between DHT11 and W5100S is straightforward. The host device sends a data request to DHT11. Then, DHT11 responds with temperature and humidity information in the form of 40 bits of digital data. Subsequently, the host device interprets this data to extract temperature and humidity values. We have implemented this process using PIO code. For a more detailed mechanism, please refer to the information below.
Through the above process, we can send the processed data to the application using MQTT communication. The image below represents the result of this project.
-
W5100S-MQTT-DHT11
The entire code, including each basic code, can be referenced at the Github link below.