iot-atmega328p-eth
minimal setup to debug atmega328p with enc28j60/w5500
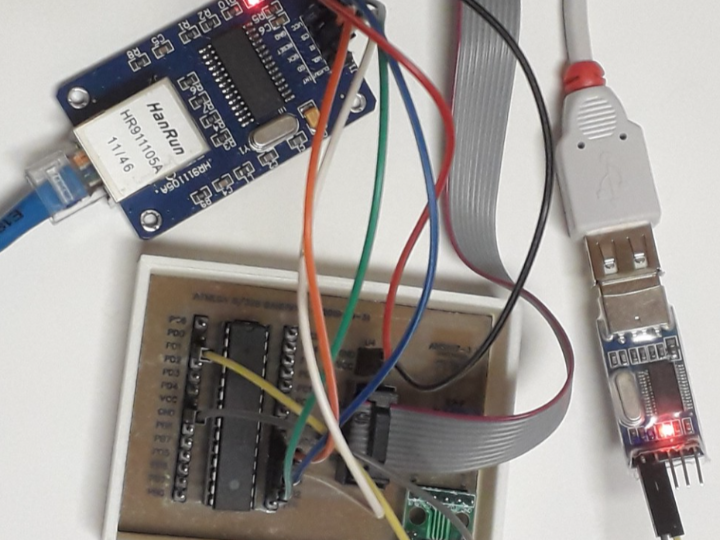
This project demonstrates setting up a minimal Internet of Things (IoT) environment using an ATmega328P microcontroller along with a W5500 Ethernet module. The provided Arduino sketch configures the microcontroller to act as a simple server, facilitating network connectivity and data exchange.
Detailed Configuration and Code Analysis
Key Components:
- ATmega328P Microcontroller: A versatile and widely-used microcontroller, ideal for numerous DIY electronics and robotics projects.
- W5500 Ethernet Module: This module offers robust network capabilities, enabling the microcontroller to communicate over a TCP/IP network.
Code Configuration:
Interface Selection:
//#define USE_ENC28J60
#define USE_W5500
This part of the code allows for the selection between two types of Ethernet interfaces—ENC28J60 and W5500. Here, the W5500 is activated to demonstrate its usage.
Network Configuration:
#define MACADDRESS 0x30, 0xca, 0x8d, 0x9f, 0x5b, 0x89
#define MYIPADDR 10, 10, 4, 110
#define MYIPMASK 255, 255, 255, 0
#define MYDNS 10, 10, 0, 6
#define MYGW 10, 10, 0, 1
#define LISTENPORT 1000
These definitions set up essential network parameters like MAC address, IP address, subnet mask, DNS, and gateway for a static IP configuration.
Implementation Details
Sketch Explanation:
Library Inclusions: Depending on the Ethernet module chosen, the appropriate library is included. For the W5500:
#include <Ethernet.h>
Server Initialization:
EthernetServer server(LISTENPORT);
An EthernetServer
object is initialized to listen on port 1000, preparing the device to handle incoming network connections.
Network Initialization in setup()
:
uint8_t mac[6] = {MACADDRESS};
uint8_t myIP[4] = {MYIPADDR};
Ethernet.begin(mac, myIP, myDNS, myGW, myMASK);
The network is configured with static IP settings to enhance control and manageability within the network.
Handling Incoming Connections in loop()
:
if (EthernetClient client = server.available()) {
while (client.available() > 0) {
// Code to handle client data
}
client.stop();
}
This segment continuously checks for and processes incoming client connections, reading messages and responding accordingly.
Usage Scenario:
- Users can connect to this server using tools like Telnet by entering the IP address and port. The simplicity of this setup is essential for debugging and developing network-related functionalities in IoT projects.
Security Considerations:
- The project lacks encrypted communications (HTTPS), which is crucial for ensuring security, especially when exposed to the internet. Using a secure proxy like Nginx to add SSL/TLS encryption is recommended.
- Further security measures such as API tokens should be considered for operations that could modify system states or control actuators.
Conclusion:
This Arduino-based project utilizing an ATmega328P and W5500 Ethernet module serves as a foundational guide to networked applications using microcontrollers. It showcases the integration of standard communication protocols with embedded systems, opening the door to more complex IoT applications that require remote monitoring and control.