Arduino-based Irrigation System Project
Arduino-based Irrigation System Project
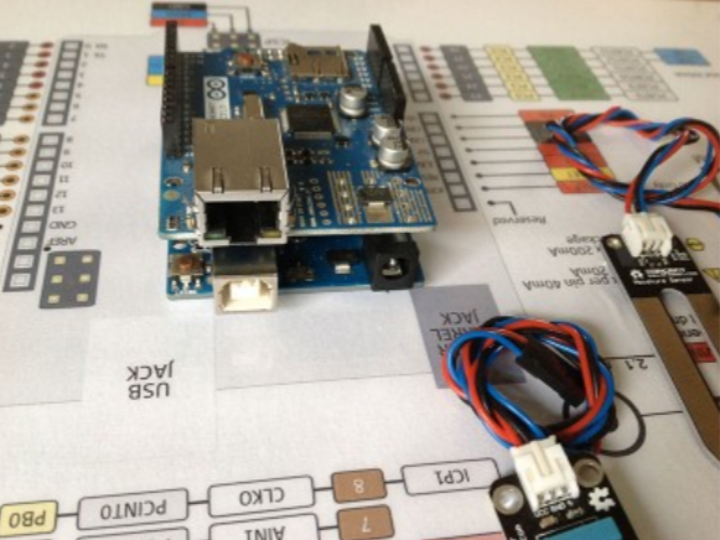
Project Overview: This project involves creating an irrigation system using Arduino, which not only automates watering but also monitors the garden's condition by recording related data to a web database. This integrated system allows for efficient water management by providing real-time feedback on the garden's status through a web browser or graphic interface.
Core Components:
- One of the following Arduino models: Uno, Due, Leonardo, Mega
- Ethernet Shield
- Ethernet cable
- Internet connection with DHCP support
- LAMP server (Linux, Apache, MySQL, PHP)
Sensors and Devices:
- Temperature and humidity sensor
- Soil moisture sensor
- Additional sensors such as light intensity, wind speed, and rainfall measurement sensors can be integrated to further enhance the system's monitoring capabilities.
Data Management:
- Collected data is stored in a MySQL database, and visualized through a web interface using PHP.
- Users can check real-time readings from each sensor via a web browser.
MySQL Database Setup:
- A table is created to store data from each sensor, along with additional information such as the IP address to manage the origin of the data.
DROP TABLE IF EXISTS `monitoraggio_irrigazione`;
CREATE TABLE IF NOT EXISTS `monitoraggio_irrigazione` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`data_time` datetime NOT NULL,
`ip_remote` varchar(16) NOT NULL,
`codice_impianto` varchar(32) NOT NULL,
`sensoreA` float DEFAULT NULL,
`sensoreB` float DEFAULT NULL,
`sensoreC` float DEFAULT NULL,
`sensoreD` float DEFAULT NULL,
`sensoreE` float DEFAULT NULL,
`sensoreF` float DEFAULT NULL,
`sensoreG` float DEFAULT NULL,
`sensoreH` float DEFAULT NULL,
`sensoreI` float DEFAULT NULL,
`sensoreL` float DEFAULT NULL,
`sensoreM` float DEFAULT NULL,
`sensoreN` float DEFAULT NULL,
`sensoreO` float DEFAULT NULL,
`sensoreP` float DEFAULT NULL,
`sensoreQ` float DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `codice_impianto` (`codice_impianto`)
) ENGINE=MyISAM DEFAULT CHARSET=utf8 COMMENT='Monitoraggio Irrigazione' AUTO_INCREMENT=1;
PHP Script:
- A PHP script configured on the server receives sensor data measured by Arduino and stores it in the database.
<?php
/*****************************************************/
/** Nome utente del database MySQL */
define('DB_USER', 'USERNAME');
/** Password del database MySQL */
define('DB_PASSWORD', 'PASSWORD');
/** Hostname MySQL */
define('DB_HOST', 'localhost');
/** DataBase MySQL */
define('DB_NAME', 'DATABASE');
/** Table MySQL */
define('DB_TABLE', 'monitoraggio_irrigazione');
/*****************************************************/
$_path_info = array();
/*****************************************************/
if ( !empty($_SERVER['PATH_INFO'])) {
$_path_info = explode( '/',$_SERVER['PATH_INFO'] );
} else {
die( "ERROR001" );
}
/*****************************************************/
$codice = $_path_info[1];
$sensoreA = $_path_info[2];
$sensoreB = $_path_info[3];
$sensoreC = $_path_info[4];
$sensoreD = $_path_info[5];
$sensoreE = $_path_info[6];
$sensoreF = $_path_info[7];
$sensoreG = $_path_info[8];
$sensoreH = $_path_info[9];
$sensoreI = $_path_info[10];
$sensoreL = $_path_info[11];
$sensoreM = $_path_info[12];
$sensoreN = $_path_info[13];
$sensoreO = $_path_info[14];
$sensoreP = $_path_info[15];
$sensoreQ = $_path_info[16];
/*****************************************************/
@mysql_connect(DB_HOST,DB_USER,DB_PASSWORD) or die( "ERROR002" );
@mysql_select_db(DB_NAME) or die( "ERROR003" );
/*****************************************************/
$sql="INSERT INTO " . DB_TABLE . "(
data_time,
ip_remote,
codice_impianto,
sensoreA,
sensoreB,
sensoreC,
sensoreD,
sensoreE,
sensoreF,
sensoreG,
sensoreH,
sensoreI,
sensoreL,
sensoreM,
sensoreN,
sensoreO,
sensoreP,
sensoreQ
) VALUES (
NOW(),
'" . $_SERVER['REMOTE_ADDR'] . "',
'" . $codice . "',
'" . $sensoreA . "',
'" . $sensoreB . "',
'" . $sensoreC . "',
'" . $sensoreD . "',
'" . $sensoreE . "',
'" . $sensoreF . "',
'" . $sensoreG . "',
'" . $sensoreH . "',
'" . $sensoreI . "',
'" . $sensoreL . "',
'" . $sensoreM . "',
'" . $sensoreN . "',
'" . $sensoreO . "',
'" . $sensoreP . "',
'" . $sensoreQ . "'
)";
@mysql_query($sql) or die( "ERROR004" );
/*****************************************************/
@mysql_close();
die( "SUCCESS" );
?>
Arduino-based Irrigation System Sketch Explanation
In this project, we utilize Arduino to construct an irrigation system that connects to a website and focuses on sending fixed values rather than measuring actual sensor data. This approach is particularly useful during the early stages of system design for testing network connectivity and data transmission logic.
/*
* Progetto Irrigazione Arduino - prima parte
*
* Autore: Alfieri Mauro
* Twitter: @mauroalfieri
*
* Tutorial su: https://www.mauroalfieri.it
*
*/
#include <SPI.h>
#include <Ethernet.h>
#define TIME_MISURA 60000
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
char server[] = "www.mauroalfieri.it";
char buffer[150];
char codiceImpianto[32] = "1234567890123456789012345678901";
int sensoreA = 0;
int sensoreB = 0;
int sensoreC = 0;
int sensoreD = 0;
int sensoreE = 0;
int sensoreF = 0;
int sensoreG = 0;
int sensoreH = 0;
int sensoreI = 0;
int sensoreL = 0;
int sensoreM = 0;
int sensoreN = 0;
int sensoreO = 0;
int sensoreP = 0;
int sensoreQ = 0;
EthernetClient client;
void setup() {
Serial.begin(9600);
while (!Serial) {
; // wait for serial port to connect. Needed for Leonardo only
}
if (Ethernet.begin(mac) == 0) {
Serial.println("Failed to configure Ethernet using DHCP");
// no point in carrying on, so do nothing forevermore:
for(;;)
;
}
delay(1000);
}
void loop() {
sensoreA = 1;
sensoreB = 1;
sensoreC = 1;
sensoreD = 1;
sensoreE = 1;
sensoreF = 1;
sensoreG = 1;
sensoreH = 1;
sensoreI = 1;
sensoreL = 1;
sensoreM = 1;
sensoreN = 1;
sensoreO = 1;
sensoreP = 1;
sensoreQ = 1;
send2server();
delay( TIME_MISURA );
}
void send2server() {
Serial.println("connecting...");
if (client.connect(server, 80)) {
Serial.println("connected");
sprintf(buffer, "%s%s%s/%s/%d/%d/%d/%d/%d/%d/%d/%d/%d/%d/%d/%d/%d/%d/%d/%d %s", "GET http://", server, "/progetto_irrigazione/saveData.php", codiceImpianto, sensoreA, sensoreB, sensoreC, sensoreD, sensoreE, sensoreF, sensoreG, sensoreH, sensoreI, sensoreL, sensoreM, sensoreN, sensoreO, sensoreP, sensoreQ, "HTTP/1.1" );
Serial.println( buffer );
client.println(buffer);
client.println();
}
while (client.available()) {
char c = client.read();
Serial.print(c);
}
if (!client.connected()) {
Serial.println();
Serial.println("disconnecting.");
client.stop();
}
}
Key Components of the Sketch:
Including Libraries
SPI.h
,Ethernet.h
: Essential Arduino libraries for network communication.- Additional libraries like
SD.h
for data logging andWire.h
for time management may be required for expanded functionalities.
Network Configuration
- MAC and server address setup: Specifies the unique MAC address for the Ethernet shield and the URL of the server to which the data will be transmitted.
Data Transmission Function (send2server)
- Sends data from Arduino to the server using an HTTP GET request. This function transmits sensor data along with an identification code to the server, allowing it to be stored in a database.
Periodic Data Transmission
- Data is sent to the server at intervals defined by the
TIME_MISURA
constant (e.g., 60 seconds), implemented using thedelay(TIME_MISURA);
function.
Server Connection and Response Handling
- Attempts to connect to the server; upon successful connection, data is transmitted, and responses from the server are received. The client is closed after the connection ends.
Potential Derivative Project Ideas:
- Smart Agriculture System: Optimize the growing conditions of crops in real-time by interfacing with various environmental sensors.
- Automated Environmental Monitoring System: Develop an integrated monitoring and control system for urban or industrial environmental management.
- Educational Experiment Kit: Use as a project in schools or educational institutions to collect and analyze real environmental data.
- Eco-Friendly Building Management System: Create an automated irrigation and environmental control system to manage indoor plants within buildings.
This project extends beyond simply automating an irrigation system to collecting and analyzing environmental data, enabling more sustainable and efficient water resource management. Its high scalability and integration capabilities with various sensors make it adaptable to other automated systems as well.