iot_based_home_automation_sol
To control home devices using Blynk IoT app through Blynk Cloud Server.
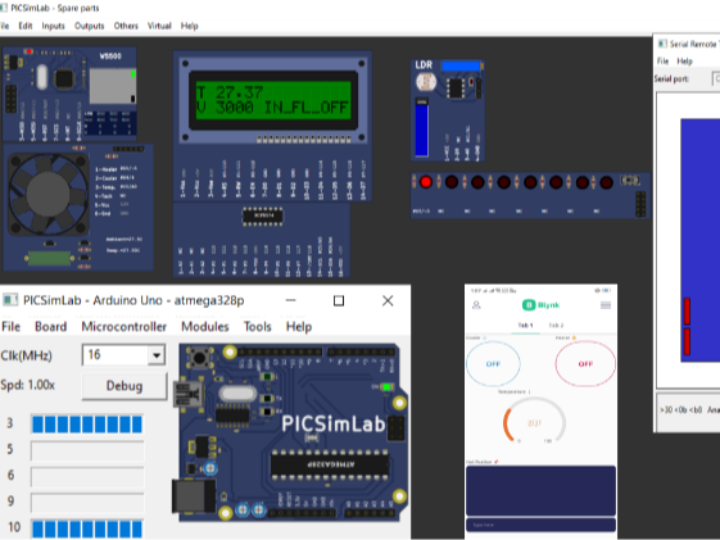
IoT Based Home Automation Solution
Project Overview: This project is focused on creating an IoT-based home automation solution that allows remote control of various home devices such as garden lights, a temperature system, and a water tank using the Blynk IoT app. The system integrates hardware components like sensors and actuators, and it is simulated using the PICSim Lab Emulator.
Tech Stack and Components:
Hardware:
- LDR Sensor: Used for detecting light intensity to control garden lights.
- LED: Acts as the garden light.
- CLCD hd 44780: A character LCD for displaying system status.
- Arduino UNO (atmega328p): The main microcontroller used for interfacing with sensors and actuators.
- Ethernet Shield (ETH w5500): Provides internet connectivity to the Arduino.
- Temperature System: Includes a heater and cooler for managing home temperature.
- Water Tank (Serial Remote Tank): For simulating a water tank's inlet and outlet control.
Software/Tools:
- Arduino IDE: For programming the Arduino board.
- PICSim Lab Emulator: For simulating the hardware setup.
- Blynk Application: Both mobile and web versions to control and monitor the system.
Languages Used:
- Embedded C/C++
Key Features and Working:
Garden Light Control:
- Goal: Lights are off during the day and on at night.
- Working: Uses an LDR sensor to measure light intensity. When the ambient light is low, resistance increases, turning the LED on. Conversely, during high light conditions, the LED turns off.
Temperature System Control:
- Goal: Remote control of heater and cooler using the Blynk app.
- Working: The system reads temperature from the sensor and displays it on the CLCD and mobile app. If the temperature exceeds 35°C while the heater is on, it automatically turns off and sends a notification to the mobile app.
Water Tank Control:
- Goal: Manage water tank levels and control inlet/outlet valves.
- Working: The system reads the tank's water volume and displays it on the CLCD and mobile app. If the water level drops below 2000L, the inlet valve turns on automatically, and a notification is sent. When the level reaches 3000L, the inlet valve turns off, and another notification is sent.
W5500 Ethernet Shield Usage:
The W5500 Ethernet Shield plays a crucial role in providing internet connectivity to the Arduino board, enabling it to communicate with the Blynk Cloud Server. Here’s how it is used in the project:
Initial Setup:
- Libraries for SPI and Ethernet are included (
SPI.h
andEthernet.h
). - The
BlynkSimpleEthernet.h
library is used to interface with the Blynk app.
Network Configuration:
- The Ethernet shield is configured to connect to the local network using DHCP or a static IP address.
- The Blynk authentication token, device name, and template ID are set up to link the hardware with the Blynk server.
Communication:
- The Ethernet shield enables the Arduino to send and receive data from the Blynk cloud.
- Sensor data (temperature, light intensity, water volume) is sent to the Blynk app.
- Control commands from the Blynk app (e.g., turning on/off the heater or cooler) are received and executed by the Arduino.
Code Structure:
home_automation_blynk_controlled.ino: Main Arduino sketch
- Setup and Definitions: Includes libraries and sets up Blynk, LCD, temperature system, LDR, and serial tank.
- Looping: Executes Blynk and timer tasks.
- Display: Updates temperature and water volume on the CLCD.
- Control Logic: Manages garden lights, temperature control, and water tank valves based on sensor readings and Blynk app inputs.
ldr.cpp and ldr.h: LDR sensor handling
- Initialization: Sets up LDR sensor pin modes.
- Brightness Control: Adjusts garden light brightness based on LDR readings.
serial_tank.cpp and serial_tank.h: Water tank control
- Serial Communication: Manages communication with the water tank system.
- Valve Control: Functions to enable or disable inlet and outlet valves.
temperature_system.cpp and temperature_system.h: Temperature system control
- Initialization: Sets up temperature sensor and control pins.
- Temperature Reading: Converts analog readings to temperature values.
- Heater/Cooler Control: Functions to control heater and cooler states.
Setting Up Blynk IoT App:
- Create Account: Register on the Blynk web version.
- Create Template: Define a template named ‘Home Automation’.
- Add Device: Link the device with the Blynk server using the template ID and auth token.
- Add Datastreams: Configure data streams for sensors and actuators.
- Setup Widgets: Add widgets in the mobile app to control and monitor the system.
- Arduino IDE Setup: Install necessary libraries, write the code, compile, and upload the HEX file to the PICSim Lab Emulator.
Demonstration:
A complete demonstration of the project is available in a YouTube video: Home Automation using Blynk and Arduino.
This project illustrates how IoT technology can be leveraged for home automation, providing convenience, efficiency, and remote monitoring/control capabilities through a user-friendly mobile interface.