LED On/Off Web Server with W55RP20
In this WCC, we will create a simple web server to turn the user LED on and off on the W55RP20-EVB-Pico.
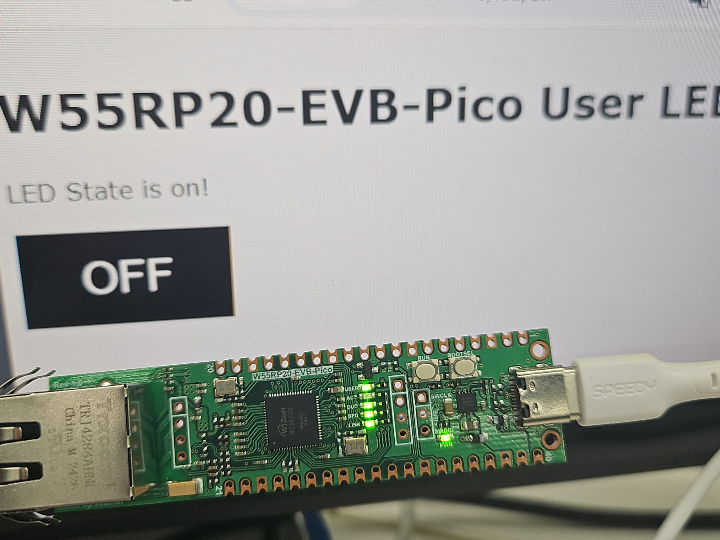
Hardware Requirements
- W55RP20-EVB-PICO
- Desktop or Laptop
- USB Type-C Cable
Software Tools
- Arduino IDE
Setup board
First, set up the W55RP20-EVB-Pico board in the Arduino IDE. Go to the 'Preferences' menu under the 'File" tab at the top.
Click the 'Additional Boards Manager URLs' button as shown in the image below and enter the following link.
https://github.com/earlephilhower/arduino-pico/releases/download/global/package_rp2040_index.json
After that, go to the 'Board' menu under the 'Tools' tab again, and you should be able to find 'Wiznet W55RP20-EVB-Pico' as shown in the image below.
If you cannot find it, it is recommended to close and reopen the Arduino IDE.
In this WCC, we will use the built-in USER LED on the W55RP20-EVB-Pico. The USER LED is connected to GPIO19, so we declare it as 19 and set its pinMode.
The web server is configured as follows: when the button is pressed, it toggles between /led/on
and /led/off
, changing the LED state accordingly.
Run
Occasionally, when uploading a program to the Pico using the Arduino IDE, you may encounter a "No drive deploy" message, causing the upload to fail.
In that case, when the output messages show either "Scanning for device" or "Uploading..." as shown in the image below, it is advisable to manually press and hold the BOOTSEL button on the W55RP20-EVB-Pico while clicking the RUN button.
When executed, it will be displayed as follows.
Code
LED_ simple_webserver.ino
#include <WiFiClient.h>
#include <WebServer.h>
#include <LEAmDNS.h>
#include <W55RP20lwIP.h>
Wiznet55rp20lwIP eth(1 /* chip select */);
WiFiServer server(80);
const int userLed = 19;
unsigned long currentTime = millis();
unsigned long previousTime = 0;
const long timeoutTime = 2000;
String header;
String picoLEDState = "off";
void setup() {
Serial.begin(115200);
delay(5000);
Serial.println();
Serial.println();
Serial.println("Starting Ethernet port");
Serial.println("....");
pinMode(userLed, OUTPUT);
digitalWrite(userLed, LOW);
// Start the Ethernet port
if (!eth.begin()) {
Serial.println("No wired Ethernet hardware detected. Check pinouts, wiring.");
while (1) {
delay(1000);
}
}
// Wait for connection
while (eth.status() != WL_CONNECTED) {
delay(500);
Serial.print(".");
}
Serial.print("IP address: ");
Serial.println(eth.localIP());
if (MDNS.begin("W55RP20")) {
Serial.println("MDNS responder started");
}
server.begin();
}
void loop() {
WiFiClient client = server.accept();
if (client) {
currentTime = millis();
previousTime = currentTime;
Serial.println("New Client.");
String currentLine = "";
while (client.connected() && currentTime - previousTime <= timeoutTime) {
currentTime = millis();
if (client.available()) {
char c = client.read();
Serial.write(c);
header += c;
if (c == '\n') {
if (currentLine.length() == 0) {
client.println("HTTP/1.1 200 OK");
client.println("Content-type:text/html");
client.println("Connection: close");
client.println();
if (header.indexOf("GET /led/on") >= 0) {
Serial.println("LED on");
picoLEDState = "on";
digitalWrite(userLed, HIGH);
} else if (header.indexOf("GET /led/off") >= 0) {
Serial.println("LED off");
picoLEDState = "off";
digitalWrite(userLed, LOW);
}
client.println("<!DOCTYPE html><html>");
client.println("<head><meta name=\"viewport\" content=\"width=device-width, initial-scale=1\">");
client.println("<link rel=\"icon\" href=\"data:,\">");
client.println("<style>html { font-family: Verdana, sans-serif; margin: 0; padding: 10px; background: #f5f5f5; color: #333; } body { max-width: 800px; margin: 0 auto; }");
client.println(".button { background-color: #ADFF2F; border: none; color: white; padding: 16px 40px;");
client.println("text-decoration: none; font-size: 30px; margin: 2px; cursor: pointer;}");
client.println(".button2 {background-color: #000000;}</style></head>");
client.println("<body><h1>W55RP20-EVB-Pico User LED</h1>");
client.println("<p>LED State is " + picoLEDState + "!" + "</p>");
if (picoLEDState == "off") {
client.println("<p><a href=\"/led/on\"><button class=\"button\">ON</button></a></p>");
} else {
client.println("<p><a href=\"/led/off\"><button class=\"button button2\">OFF</button></a></p>");
}
client.println("</body></html>");
client.println();
break;
} else {
currentLine = "";
}
} else if (c != '\r') {
currentLine += c;
}
}
}
header = "";
client.stop();
Serial.println("Client disconnected.");
Serial.println("");
}
}