WizAir - Indoor Air Quality Monitoring and Alert System
WizAir is an indoor Air quality monitoring and alert system, It uses WizFi360-EVB-Pico with BEM680 Environmental Sensor.
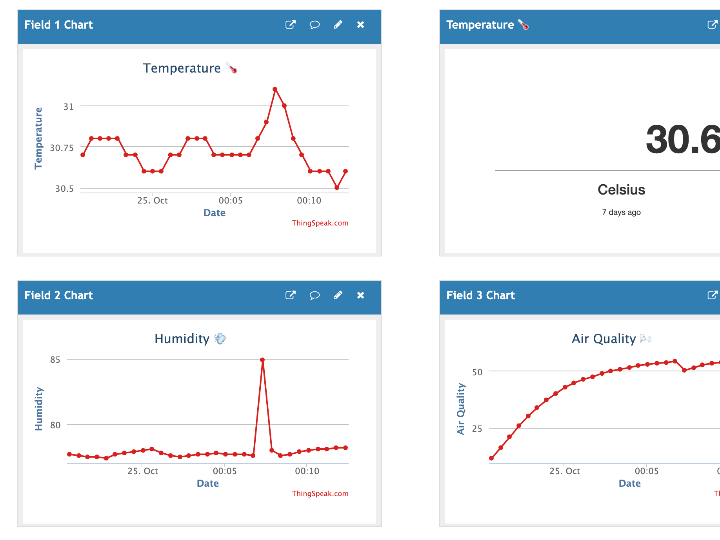
Software Apps and online services
ThingSpeak IoT - ThingView - ThingSpeak viewer
x 1
WizAir - Indoor Air Quality Monitoring and Alert System 💨
This is Proof of the Concept of an air quality monitoring and alert system, which can display the current air quality and alert when the quality of air passes a certain limit.
Things Used 🛠
WizFi360-EVB-Pico : The WizFi360-EVB-Pico is based on Raspberry Pi RP2040 and adds Wi-Fi connectivity using WizFi360. It is pin compatible with the Raspberry Pi Pico board and can be used for IoT Solution development. It is used as the main controller to read the sensor data from the sensor and publish it to the IoT cloud via WiFi.
BME680 Sensor : The BME680 is the first gas sensor that integrates high-linearity and high-accuracy gas, pressure, humidity and temperature sensors. It is specially developed for mobile applications and wearables where size and low power consumption are critical requirements. The BME680 guarantees - depending on the specific operating mode - optimized consumption, long-term stability and high EMC robustness. In order to measure air quality for personal well-being the gas sensor within the BME680 can detect a broad range of gases such as volatile organic compounds (VOC). You can find more details here https://www.bosch-sensortec.com/products/environmental-sensors/gas-sensors/bme680/
Thingspeak IoT Cloud : ThingSpeak is an IoT analytics platform service that allows you to aggregate, visualize, and analyze live data streams in the cloud. More details https://thingspeak.com/ .
Architecture 🗞
Connection Diagram ⛓
The connection Diagram is very simple where
| BME680 Sensor | WizFi360-EVB-Pico|
----------------------------------------------------------------
3.3v VCC | 3.3v VCC on Pin36
GND | GND on Pin38
SDA | GPIO 8/Pin11
SCL | GPIO 9/Pin12
Code 💻
#include <stdlib.h>
#include <Wire.h>
#include <SPI.h>
#include <Adafruit_Sensor.h>
#include "Adafruit_BME680.h"
#include "WizFi360.h"
// setup according to the device you use
#define WIZFI360_EVB_PICO
//// Emulate Serial1 on pins 6/7 if not present
#ifndef HAVE_HWSERIAL1
#include "SoftwareSerial.h"
#if defined(ARDUINO_MEGA_2560)
SoftwareSerial Serial1(6, 7); // RX, TX
#elif defined(WIZFI360_EVB_PICO)
SoftwareSerial Serial2(6, 7); // RX, TX
#endif
#endif
///* Baudrate */
#define SERIAL_BAUDRATE 115200
#if defined(ARDUINO_MEGA_2560)
#define SERIAL1_BAUDRATE 115200
#elif defined(WIZFI360_EVB_PICO)
#define SERIAL2_BAUDRATE 115200
#endif
#define SEALEVELPRESSURE_HPA (1013.25)
Adafruit_BME680 bme; // I2C
/* Wi-Fi info */
char ssid[] = "WiFi SSID Name"; // your network SSID (name)
char pass[] = "WiFi Password"; // your network password
int status = WL_IDLE_STATUS; // the Wifi radio's status
char server[] = "api.thingspeak.com"; // server address
String apiKey = "Thingsspeak API"; // apki key
// sensor buffer
char temp_buf[10];
char humi_buf[10];
char airQuality[10];
char pressure[10];
char altitudes[10];
char pres_buf[10];
char gas_buf[10];
char alti_buf[10];
unsigned long lastConnectionTime = 0; // last time you connected to the server, in milliseconds
const unsigned long postingInterval = 30000L; // delay between updates, in milliseconds
// Initialize the Ethernet client object
WiFiClient client;
void setup() {
// Set up oversampling and filter initialization
bme.setTemperatureOversampling(BME680_OS_8X);
bme.setHumidityOversampling(BME680_OS_2X);
bme.setPressureOversampling(BME680_OS_4X);
bme.setIIRFilterSize(BME680_FILTER_SIZE_3);
bme.setGasHeater(320, 150); // 320*C for 150 ms
// initialize serial for debugging
Serial.begin(SERIAL_BAUDRATE);
// initialize serial for WizFi360 module
#if defined(ARDUINO_MEGA_2560)
Serial1.begin(SERIAL1_BAUDRATE);
#elif defined(WIZFI360_EVB_PICO)
Serial2.begin(SERIAL2_BAUDRATE);
#endif
// initialize WizFi360 module
#if defined(ARDUINO_MEGA_2560)
WiFi.init(&Serial1);
#elif defined(WIZFI360_EVB_PICO)
WiFi.init(&Serial2);
#endif
if (!bme.begin()) {
Serial.println("Could not find a valid BME680 sensor, check wiring!");
while (1);
}
// check for the presence of the shield
if (WiFi.status() == WL_NO_SHIELD) {
Serial.println("WiFi shield not present");
// don't continue
while (true);
}
// attempt to connect to WiFi network
while ( status != WL_CONNECTED) {
Serial.print("Attempting to connect to WPA SSID: ");
Serial.println(ssid);
// Connect to WPA/WPA2 network
status = WiFi.begin(ssid, pass);
}
Serial.println("You're connected to the network");
// printWifiStatus();
Serial.println("Startings");
//First transmitting
sensorRead();
thingspeakTrans();
}
void loop() {
// if there's incoming data from the net connection send it out the serial port
// this is for debugging purposes only
while (client.available()) {
char c = client.read();
Serial.print("recv data: ");
Serial.write(c);
Serial.println();
}
// if 30 seconds have passed since your last connection,
// then connect again and send data
if (millis() - lastConnectionTime > postingInterval) {
sensorRead();
thingspeakTrans();
}
}
// Read sendsor value
void sensorRead() {
if (! bme.performReading()) {
Serial.println("Failed to perform reading :(");
return;
}
Serial.print("Temperature = ");
Serial.print(bme.temperature);
Serial.println(" *C");
String strTemp = dtostrf(bme.temperature, 4, 1, temp_buf);
Serial.print("Pressure = ");
Serial.print(bme.pressure / 100.0);
Serial.println(" hPa");
String strPres = dtostrf(bme.pressure / 100.0, 4, 1, pres_buf);
Serial.print("Humidity = ");
Serial.print(bme.humidity);
Serial.println(" %");
String strHumi = dtostrf(bme.humidity, 4, 1, humi_buf);
Serial.print("Gas = ");
Serial.print(bme.gas_resistance / 1000.0);
Serial.println(" KOhms");
String strGas = dtostrf(bme.gas_resistance / 1000.0, 4, 1, gas_buf);
Serial.print("Approx. Altitude = ");
Serial.print(bme.readAltitude(SEALEVELPRESSURE_HPA));
Serial.println(" m");
String strAlti = dtostrf(bme.readAltitude(SEALEVELPRESSURE_HPA), 4, 1, alti_buf);
Serial.println();
delay(2000);
}
//Transmitting sensor value to thingspeak
void thingspeakTrans() {
// close any connection before send a new request
// this will free the socket on the WiFi shield
client.stop();
// if there's a successful connection
if (client.connect(server, 80)) {
Serial.println("Connecting...");
// send the Get request
client.print(F("GET /update?api_key="));
client.print(apiKey);
client.print(F("&field1="));
client.print(temp_buf);
client.print(F("&field2="));
client.print(humi_buf);
client.print(F("&field3="));
client.print(gas_buf);
client.println();
// note the time that the connection was made
lastConnectionTime = millis();
}
else {
// if you couldn't make a connection
Serial.println("Connection failed");
}
}
void printWifiStatus() {
// print the SSID of the network you're attached to
Serial.print("SSID: ");
Serial.println(WiFi.SSID());
// print your WiFi shield's IP address
IPAddress ip = WiFi.localIP();
Serial.print("IP Address: ");
Serial.println(ip);
// print the received signal strength
long rssi = WiFi.RSSI();
Serial.print("Signal strength (RSSI):");
Serial.print(rssi);
Serial.println(" dBm");
}
Output 👀
Next Step 📍
- Create a custom PCB
- Design and 3D Print an enclosure.
Thank You wiznet for proving the fee development kit and proving the examples and documentation. 🙌