CircuitPython MQTT Example with W5500-EVB-Pico2
A simple MQTT example using W5500-EVB-Pico2 with CircuitPython. Connect via Ethernet, send/receive messages, and control the LED by topic.
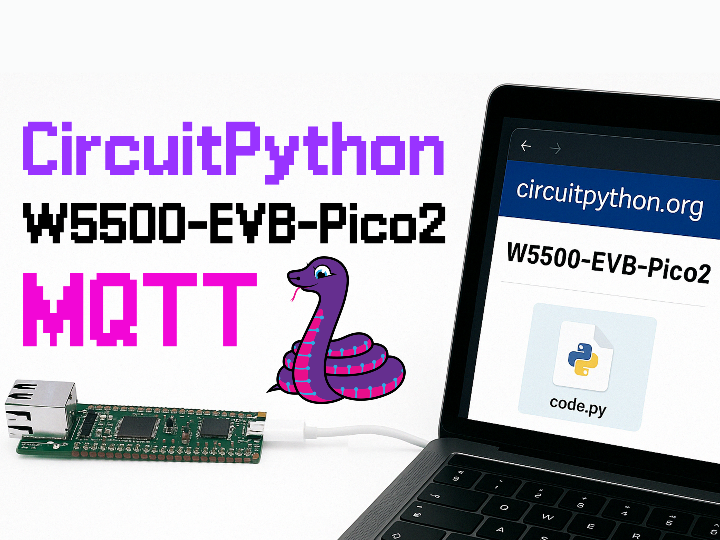
Environment
Board: W5500-EVB-Pico2
CircuitPython Version: 10.0.0-alpha.2
MQTT Broker: Local Mosquitto (with allow_anonymous enabled)
Libraries Used:
adafruit_wiznet5k
adafruit_minimqtt
The board was connected to the network via Ethernet using a static IP, and the Mosquitto broker was hosted on a local PC within the same network.
Example Highlights
- Static IP and client configuration
eth.ifconfig = (
(192, 168, 11, 50), # Static IP address
(255, 255, 255, 0), # Subnet mask
(192, 168, 11, 1), # Gateway
(8, 8, 8, 8) # DNS server
)
mqtt_client = MQTT.MQTT(
broker="192.168.11.2",
port=1883,
client_id="W5500-EVB-Pico2",
socket_pool=pool
)
- MQTT publish on topic
grace/test
msg = "on"
mqtt_client.publish("grace/test", msg)
- LED control via subscribed messages
The board periodically publishes LED state, and listens for incoming "on"/"off" commands to control the onboard LED.
What This Example Does
Subscribes to the topic grace/test
Turns on the onboard LED when receiving a message containing "on"
, and turns it off on "off"
Periodically publishes its status (either "on" or "off") to the same topic
Note: In order for
adafruit_minimqtt
to properly receive messages,mqtt_client.loop()
must be called regularly.
Without this, message callbacks will not be triggered even if messages are successfully received.
Below is the serial console output when the example code runs successfully.
The board connects to the network with IP 192.168.11.50
, connects to the Mosquitto broker, and subscribes to the topic "grace/subs"
.
It then publishes "on"
and "off"
messages to the "grace/test"
topic.
When a message is published externally to "grace/subs"
, it is received and printed to the console, and the onboard LED is controlled accordingly.
The MQTT broker accepted connections and message exchanges successfully.
For this test, we enabled anonymous access by setting allow_anonymous=true
in the Mosquitto .conf
file.
Below is the complete code.
import sys
import time
import board
import busio
import digitalio
import adafruit_minimqtt.adafruit_minimqtt as MQTT
from adafruit_wiznet5k.adafruit_wiznet5k import WIZNET5K
import adafruit_wiznet5k.adafruit_wiznet5k_socketpool as socketpool
# Set up the Ethernet SPI interface and CS pin
cs = digitalio.DigitalInOut(board.W5K_CS)
spi = busio.SPI(board.W5K_SCK, board.W5K_MOSI, board.W5K_MISO)
eth = WIZNET5K(spi, cs, is_dhcp=False)
# Manually assign static IP
eth.ifconfig = (
(192, 168, 11, 50),
(255, 255, 255, 0),
(192, 168, 11, 1),
(168, 126, 63, 1)
)
print("IP address:", eth.pretty_ip(eth.ifconfig[0]))
# Set socket handler for MQTT
pool = socketpool.SocketPool(eth)
# Set up MQTT client
mqtt_client = MQTT.MQTT(
broker="192.168.11.2",
port=1883,
client_id="W5500-EVB-Pico2",
socket_pool=pool
)
# MQTT message received callback
def message(client, topic, msg):
print("========================================")
print(f"[RECEIVED] Topic: {topic}")
print(f"[RECEIVED] Payload: {msg}")
print("========================================")
if "on" in msg.lower():
led.value = True
print("[ACTION] LED turned ON by MQTT")
elif "off" in msg.lower():
led.value = False
print("[ACTION] LED turned OFF by MQTT")
# MQTT connect callback
def connected(client, userdata, flags, rc):
print("[INFO] Connected to MQTT broker")
client.subscribe("grace/subs")
print("[INFO] Subscribed to topic: grace/subs")
mqtt_client.on_connect = connected
mqtt_client.on_message = message
try:
mqtt_client.connect()
except Exception as e:
print("[ERROR] Failed to connect to MQTT broker.")
print("Reason:", e)
sys.exit(1)
# Set up onboard LED
led = digitalio.DigitalInOut(board.LED)
led.direction = digitalio.Direction.OUTPUT
# Loop: LED blinking and MQTT publish
while True:
mqtt_client.loop()
led.value = True
msg = f"on"
print(f"[PUBLISH] {msg}")
mqtt_client.publish("grace/test", msg)
time.sleep(2)
led.value = False
msg = f"off"
print(f"[PUBLISH] {msg}")
mqtt_client.publish("grace/test", msg)
time.sleep(2)
This example demonstrates that the W5500-EVB-Pico2 works reliably for network communication in a CircuitPython environment.
It's easy to expand this setup for use with sensor data publishing, device coordination, or cloud service integration.
We appreciate your interest in the board’s official support and the related pull request.